Python Function Show N Fibonacci Terms – You can write a Python program to print the first n terms of the Fibonacci series using a loop. Here’s a simple example using a for loop:
Source Code
def fibonacci(n): fib_series = [] a, b = 0, 1 for _ in range(n): fib_series.append(a) a, b = b, a + b return fib_series n = int(input("Enter the number of terms for the Fibonacci series: ")) if n <= 0: print("Please enter a positive integer.") else: result = fibonacci(n) print("Fibonacci series with", n, "terms:") print(result)
Output
Enter the number of terms for the Fibonacci series: 5
Fibonacci series with 5 terms:
[0, 1, 1, 2, 3]
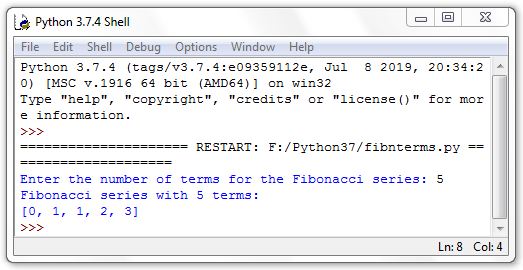
Let me explain the code step by step:
We start by defining a function called fibonacci that takes one argument, n, which represents the number of terms to generate in the Fibonacci series. This function will return a list containing the Fibonacci series.
We initialize an empty list fib_series to store the Fibonacci series and two variables a and b to keep track of the current and next Fibonacci numbers. Initially, a is set to 0 and b is set to 1, which are the first two numbers in the series.
We use a for loop to generate the Fibonacci series. The loop runs n times, where n is the number of terms requested by the user.
Inside the loop, we append the current Fibonacci number a to the fib_series list. This adds the current Fibonacci number to our series.
We then update a and b to calculate the next Fibonacci number in the sequence. The Fibonacci series is generated by adding the current number a and the next number b to get the new value of a, and assigning the current value of b to a.
After the loop has finished running, the fib_series list contains the first n terms of the Fibonacci series.
The program then takes user input to specify the number of terms (n) they want to print.
It checks if the user input is a positive integer. If n is less than or equal to 0, it displays an error message. Otherwise, it proceeds to generate and print the Fibonacci series with the requested number of terms.
Finally, the generated Fibonacci series is printed to the console.
This program effectively generates and prints the first n terms of the Fibonacci series using a for loop and a list to store the series.