ListBox Add Remove Move Items Visual Programming – C Sharp Windows Forms Application with:
Buttons to Add item,
Remove selected item,
View Selected Item,
Move selcted item of listbox 1 to list box 2,
Move all items of listbox 1 to list box 2
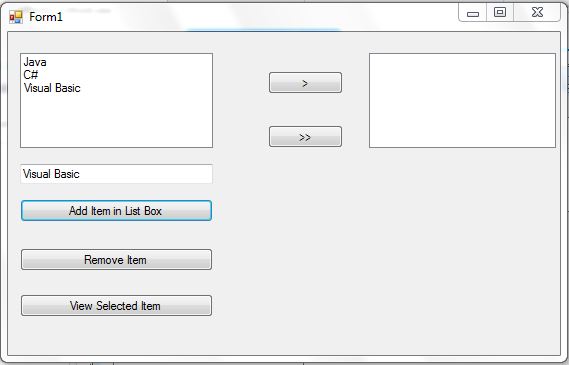
ListBox Add items, remove item, Move selcted item of listbox 1 to list box 2
using System; //using System.Collections.Generic; //using System.ComponentModel; //using System.Data; //using System.Drawing; //using System.Linq; //using System.Text; //using System.Threading.Tasks; using System.Windows.Forms; namespace ListBox2 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { listBox1.Items.Add(textBox1.Text); } private void button2_Click(object sender, EventArgs e) { listBox1.Items.Remove(listBox1.Text); } private void button3_Click(object sender, EventArgs e) { MessageBox.Show(listBox1.Text); } private void button4_Click(object sender, EventArgs e) { listBox2.Items.Add(listBox1.Text); } private void button5_Click(object sender, EventArgs e) { foreach (String item in listBox1.Items) listBox2.Items.Add(item); } } }
Explanation of Code
This code is for a Windows Forms application in C# that involves a graphical user interface (GUI) with a ListBox control and several buttons. Here’s a breakdown of the code:
Using Directives:
using System;
using System.Windows.Forms;
These lines include the necessary namespaces for the code to access classes and types defined in the System and System.Windows.Forms namespaces.
Namespace Declaration:
namespace ListBox2
{
// Class code here
}
The code is organized within the ListBox2 namespace to avoid naming conflicts.
Form Class:
public partial class Form1 : Form
{
// Constructor
public Form1()
{
InitializeComponent();
}
// Event handler methods
// …
}
This defines a class named Form1 that inherits from the Form class, which is the base class for creating Windows Forms. The constructor (public Form1()) initializes the components on the form using InitializeComponent().
Button Click Event Handlers:
private void button1_Click(object sender, EventArgs e)
{
listBox1.Items.Add(textBox1.Text);
}
private void button2_Click(object sender, EventArgs e)
{
listBox1.Items.Remove(listBox1.Text);
}
private void button3_Click(object sender, EventArgs e)
{
MessageBox.Show(listBox1.Text);
}
Button Click Event Handlers:
These methods are event handlers for the button clicks. When a button is clicked, the corresponding method is executed.
button1_Click
: Adds the text fromtextBox1
tolistBox1
.button2_Click
: Removes the selected text fromlistBox1
.button3_Click
: Shows a message box with the selected text fromlistBox1
.button4_Click
: Adds the selected text fromlistBox1
tolistBox2
.button5_Click
: Copies all items fromlistBox1
tolistBox2
.