List Box Example Program Add Remove Clear Items –
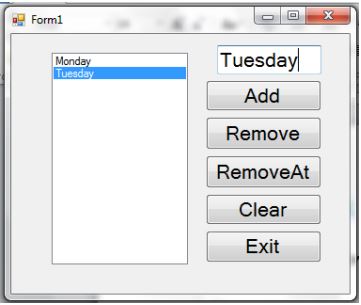
List Box Example Program Add Remove Clear Items
ListBox and Its Important Methods Used In this Windows Forms Application
In C#, a ListBox is a commonly used graphical control element that displays a list of items from which the user can select one or more. The ListBox class provides several methods to manipulate the items within it. Here’s an explanation of the `Add`, `Remove`, `RemoveAt`, and `Clear` methods of the ListBox class:
1. Add Method
The `Add` method is used to add an item to the ListBox. You can add items as strings, objects, or any other data type that can be represented as a string.
listBox1.Items.Add(“Item 1”); // Adding a string item
listBox1.Items.Add(42); // Adding an integer item
2. Remove Method
The `Remove` method is used to remove a specific item from the ListBox based on its value. It searches for the first occurrence of the specified item and removes it.
listBox1.Items.Remove(“Item 1”); // Removes the first occurrence of “Item 1”
listBox1.Items.Remove(42); // Removes the first occurrence of the integer 42
If the item is not found in the ListBox, nothing happens (no exception is thrown).
3. RemoveAt Method
The `RemoveAt` method is used to remove an item at a specific index in the ListBox. The index is zero-based, meaning the first item is at index 0, the second item at index 1, and so on.
listBox1.Items.RemoveAt(0); // Removes the first item in the ListBox
listBox1.Items.RemoveAt(2); // Removes the third item in the ListBox
If the index is out of the valid range of indices for the ListBox, an `ArgumentOutOfRangeException` exception is thrown.
4. Clear Method
The `Clear` method is used to remove all items from the ListBox.
listBox1.Items.Clear(); // Removes all items from the ListBox
Source Code
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace ListBox1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { listBox1.Items.Add(textBox1.Text); } private void button2_Click(object sender, EventArgs e) { listBox1.Items.Remove(textBox1.Text); } private void button5_Click(object sender, EventArgs e) { int i; i = int.Parse(textBox1.Text); listBox1.Items.RemoveAt(i); } private void button3_Click(object sender, EventArgs e) { listBox1.Items.Clear(); } private void button4_Click(object sender, EventArgs e) { this.Close(); } } }
hh