C Program Find Sum of Digits of N
This C language program will input a number n from the user. It will use the divide the number by 10 logic and remainder logic to find the sum of all the digits of that number provided by the user.
How Sum of Digits program works?
Let n = 123
Divide q = n /10, so q = 123 /10, hence quotient q =12.
Divide 123 by 10 for remainder now, 123 % 10 will give 3.
We know that 3 is the last digit of the entered number 123. Add 3 to sum.
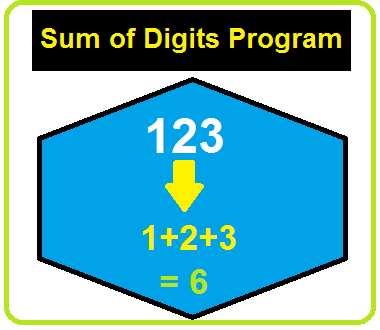
Next time we will divide 12 by 10. This will give quotient=1 and remainder =2. Add 2 to sum.
At the end we will divide 1 by 10 going quotient = 0 and remainder=1. Add 1 to sum. This will give the sum of all digits of the number 123. 1+2+3 is 6.
/* C program to find sum of digits of a number www.easycodebook.com */ #include <stdio.h> int main() { int n, num, sum = 0, digit; printf("Enter a number to find sum of its digits\n"); scanf("%d", &n); num = n; while (num != 0) { digit = num % 10; sum = sum + digit; num = num / 10; } printf("Sum of digits of %d = %d\n", n, sum); return 0; }
Output
Enter a number to find sum of its digits
786
Sum of digits of 786 = 21