C Program Add Two Matrix
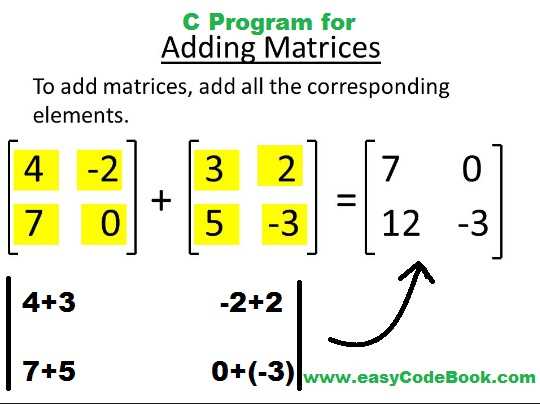
/* C program to add two matrices www.easycodebook.com */ #include <stdio.h> int main() { int m, n, i, j, matrix1[10][10], matrix2[10][10], sum[10][10]; printf("Enter the number of rows and columns of matrix\n"); scanf("%d%d", &m, &n); printf("Please Enter %d elements of first matrix\n",m*n); for (i = 0; i < m; i++) for (j = 0; j < n; j++) scanf("%d", &matrix1[i][j]); printf("Please Enter the %d elements of second matrix\n",m*n); for (i = 0; i < m; i++) for (j = 0 ; j < n; j++) scanf("%d", &matrix2[i][j]); printf("Sum of the two matrices is as follows:\n"); for (i = 0; i < m; i++) { for (j = 0 ; j < n; j++) { sum[i][j] = matrix1[i][j] + matrix2[i][j]; printf("%d\t", sum[i][j]); } printf("\n"); } return 0; }
Output
Enter the number of rows and columns of matrix
3
3
Please Enter 9 elements of first matrix
1
2
3
4
5
6
7
8
9
Please Enter the 9 elements of second matrix
5
6
4
3
2
1
7
8
9
Sum of the two matrices is as follows:
6 8 7 7 7 7 14 16 18
Rules for Matrix Addition
We can add two matrices, if they are both of the same order. That is they have same number of rows and columns.
We cannot add two matrices with different dimentions.