Python print function – Printing Output – This Python tutorial will explain the use of Python print() function for showing the output on screen in different ways.
What is print() function in Python
Python print() function is used as an output statement in Python programs.
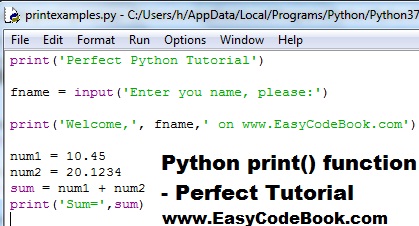
Example of print() function
Here is a simple example:
print( ‘ Welcome in Perfect Python Tutorial’ )
The above function will show a message Welcome in Perfect Python Tutorial on the screen.
Python print function – Printing Output
The General Form of print function with Examples
Syntax: A commonly used general form of print()
print(value(s), sep= ‘ ‘, end = ‘\n’)
Here value(s) represents one or more values to be printed. These can be literals or variables.
Two Optional Arguments of print() function
A Separator argument is represented by sep=’ ‘. It is optional argument of print() function. If we do not use, the default separator is a single space.
If we use print(‘Hello’,’World) , it will print
Hello World
Note: it prints two string literal values separated by default space.
But if we use print(‘Hello’,’World’, sep=’:’)
It will print using a ‘:’ separator:
Hello:World
Another Optional argument ‘end’
There is another optional argument called end. The default value of this argument is end=’\n’. Therefore, after every print() function a new line is inserted in the output.
How to use end argument in print function to stop Next Line Advancing
We can use the ‘end’ argument to keep the print function from advancing to the next line. For example:
print( 'Hello', end= ' ' ) print( 'World') Now the output will be: Hello World Note: This is because we have said by writing end=' ' that there will be a space after printing by the print function. Hence, the output is on the same line after one space. But if we have used: print('Hello') print('World') Then output will be on two lines, because default use of end argument is end='\n': Hello World
If we use end argument as end='' then there will be no space between values, as follows
print( 'Hello', end= '' )
print( 'World')
will give the output:
HelloWorld
Differnt Uses of Python print function with Examples
1. print(‘a string message’)
This form shows the given message in quotes on the screen.
2. print(variable_name)
This form shows the value of variable on the screen. For example,
num = 100
print(num)
will show 100 on screen, which is the value of num variable. We will not use quotes around variable names when printing values.
3. print(‘string message’,variable_name)
example: print(‘Sum=’,sum)
n above print() function we provide a string in quotes ‘Sum=’ which is printed as it is. We also use a variable name after a comma. Therefore, the value of variable sum will show here. Foor example:
num1=10
num2=20
sum=num1+num2
print(‘Sum=’,sum)
Now print() function will show Sum=30.
4. print() function and Next Line
The print function will automatically advance to the next line. For example, if we write the following two statements using a print() function:
print( 'Line # 1') print( 'Line # 2')
The out put will be on two lines. The first print() function will print ‘Line # 1’ and then advances to next line. Hence the second print() function prints ‘Line # 2’ on the second line. Therefore, the output will be as follows:
Line # 1 Line # 2
5. Printing Many arguments with default single space
When you use many different arguments in a print() function, it inserts a space between each arguments while displaying.
For example:
num1 = 100 num2 = 200 sum = num1 + num2 print('Sum of',n1,'and',num2,'is:',sum)
The output will consist of 6 arguments values with a single space between them.
Sum of 100 and 200 is: 300
The Sample Python Program on Using print() function
print('Perfect Python Tutorial') fname = input('Enter you name, please:') print('Welcome,', fname,' on www.EasyCodeBook.com') num1 = 10.45 num2 = 20.1234 sum = num1 + num2 print('Sum=',sum)
The ouput is: Perfect Python Tutorial Enter you name, please:Jameel Welcome, Jameel on www.EasyCodeBook.com Sum= 30.5734
Printing a nice multiple line output with print() function
if we write a print() function using triple single quotes as shown in the following, example. The output will be in multiple lines and is shown as typed in the editor.
Example:
print(''' 'Hello','world' =============== | | | | | | =============== ''')
the output will be:
'Hello','world'
===============
| |
| |
| |
===============
Similarly, consider another example:
print('''
==========================
| |
| www.EasyCodeBook.com |
| |
==========================
''')
The output will be as follows:
==========================
| |
| www.EasyCodeBook.com |
| |
==========================
How to display the output say the Words on different lines using Single print function
You can use sep=’\n’ to indicate that separator of the arguments is a new line character. Therefore, each argument in print function will appear on a new line as shown below:
The Python code: print('Hello','World', sep='\n') The output: Hello World
Pingback: Python Program Fibonacci Series Function | EasyCodeBook.com
great put up, very informative. I wonder why the other experts of this sector do not notice this. You should continue your writing. I am sure, you have a great readers’ base already!