What is the Definition of a Perfect Number in Mathematics
Example of Perfect Number
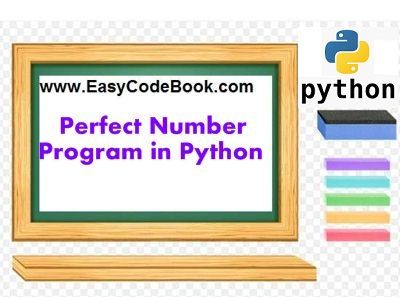
Another Definition of Perfect Number
Perfect number is a positive integer that is equal to the sum of its proper divisors. A proper divisor is a divisor other than the number itself. For example, the divisors of 6 are 1, 2,3 and 6. But the proper divisors of 6 are 1, 2 and 3. The smallest perfect number is 6, which is the sum of 1, 2, and 3. The examples of some other perfect numbers are 28, 496, and 8,128. It also means that perfect numbers are very rare.
Examples of Perfect Numbers with the Sum of their Proper Divisors
6 is a Perfect Number:
The divisors of 6 are 1, 2, 3 and 6.
The sum of the proper divisors is 1 + 2 + 3 = 6
28 is a Perfect number:
The divisors of 28 are 1, 2, 4, 7, 14 and 28.
The sum of the proper divisors is 1 + 2 + 4 + 7 + 14 = 28
496 is a Perfect number:
The divisors of 496 are 1, 2, 4, 8, 16, 31, 62, 124, 248 and 496.
The sum of the proper divisors is 1 + 2 + 4 + 8 + 16 + 31 + 62 + 124 + 248 = 496
8128 is a Perfect Number
The divisors of 8128 are 1, 2, 4, 8, 16, 32, 64, 127, 254, 508, 1016, 2032, 4064 and 8128.
The sum of the proper divisors is 1+2+4+8+16+32+64+127+254+508+1016+2032+4064 =8128.
What is the Logic of Perfect Number Program in Python
Consider the positive integer number 6.
We have to find proper divisors of 6. We know that proper divisors of 6 will range from 1 to 3(the half of 6).
Therefore, we will use a loop to get the proper divisors of six and calculate their sum. This loop will start from 1 and the final value of the loop control variable should be 3 which is half of the given number.
The Source code of Perfect Number Program in Python
# Write a Python Perfect Number program # that asks the user to enter a number. # The program should check and display # whether it is a Perfect Number. # Author: www.EasyCodeBook.com (c) num = int(input('Enter a Positive number to check for Perfect Number:')) sum = 0 print('The proper divisors of',num,'are:', end='') for i in range(1,(num//2)+1): if num%i == 0: sum = sum + i print(i,',', end='') print() print('The sum of the proper divisors of',num,'is=',sum) if num==sum: print('Therefore',num,'is a Perfect Number.') else: print('Therefore',num,'is not a Perfect Number.')
The output of Perfect Number Program
Enter a Positive number to check for Perfect Number:812 The proper divisors of 812 are:1 ,2 ,4 ,7 ,14 ,28 ,29 ,58 ,116 ,203 ,406 , The sum of the proper divisors of 812 is= 868 Therefore 812 is not a Perfect Number. Another sample output is: Enter a Positive number to check for Perfect Number:8128 The proper divisors of 8128 are:1 ,2 ,4 ,8 ,16 ,32 ,64 ,127 ,254 ,508 ,1016 ,2032 ,4064 , The sum of the proper divisors of 8128 is= 8128 Therefore 8128 is a Perfect Number. Another sample run: Enter a Positive number to check for Perfect Number:496 The proper divisors of 496 are:1 ,2 ,4 ,8 ,16 ,31 ,62 ,124 ,248 , The sum of the proper divisors of 496 is= 496 Therefore 496 is a Perfect Number.
Example of Checking a Big Number for Perfect Number Program
Enter a Positive number to check for Perfect Number:33550336 The proper divisors of 33550336 are:1 ,2 ,4 ,8 ,16 ,32 ,64 ,128 ,256 ,512 ,1024 ,2048 ,4096 ,8191 ,16382 ,32764 ,65528 ,131056 ,262112 ,524224 ,1048448 ,2096896 ,4193792 ,8387584 ,16775168 , The sum of the proper divisors of 33550336 is= 33550336 Therefore 33550336 is a Perfect Number.