Task: Write a C++ – CPP Program to write / add records in Binary File.
How this Program Works?
This C++ Program will open a binary file either by creating a new file or by just opening it if it already exists. Then it will use write() method to write for first time or append more records in the existing file. It is because we have opened the file in append mode as follows:
//opening binary file in appending / writing mode
file1.open(“d://cppfile.dat”,ios::binary |ios::app);
The following figure shows that the file cpppfile.dat has been created on D drive after execution f the C++ program.
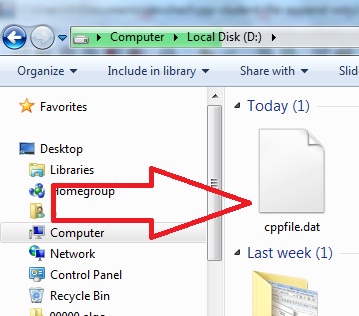
First of all a user will input a record and it is written into binary file using write()method as follows:
/* write a record to binary file */
file1.write((char*)&srecord,sizeof(srecord));
Then program will ask from the user: Add more records? press y or n:
The user will press ‘y’ if he wishes to add more records. Otherwise he will type ‘n’, if he wishes to stop the program. This input logic is maintained by a while loop.
Source code for the C ++ program to enter records in a binary file.
/* Write a C Program to add more records at end of already existing binary file that is append file operation */ #include<iostream> #include<fstream> #include<conio.h> #include<stdlib.h> #include<string> using namespace std; struct student { int rollno; char name[30]; }srecord; fstream file1; int main() { char ans; int i; //opening binary file in appending / writing mode file1.open("d://cppfile.dat",ios::binary |ios::app); if(!file1) { cout<<"File could not open"; exit(0); } while(1) { cout<<"\nEnter Student Record\n"; cout<<"Roll No:"; cin>>srecord.rollno; getchar(); cout<<"Name:"; gets(srecord.name); /* write a record to binary file */ file1.write((char*)&srecord,sizeof(srecord)); cout<<"\nRecord has been added successfully"; cout<<"\nAdd more records? press y or n ="; ans=getche(); if(ans=='n' || ans=='N') break; } file1.close(); cout<<"\nProgram ended: C++ Program To Add more Student Records in Binary File:"; return 0; }
A Sample Run of the C++ Program: write / add records in Binary File
Enter Student Record Roll No:101 Name:sajid Record has been added successfully Add more records? press y or n =y Enter Student Record Roll No:102 Name:majid Record has been added successfully Add more records? press y or n =n Program ended: C++ Program To Add more Student Records in Binary File: -------------------------------- Process exited after 41.99 seconds with return value 0 Press any key to continue . . .
Pingback: Flowcharts With Examples and Explanation of Symbols | EasyCodeBook.com