“C Program using add function” is a C language program to learn the use of function in C language. A function called addition( ) is used to calculate addition of two numbers. The main function will send two numbers to the addition function. The addition() function will add them and prints result. The user defined function has a void return type. It means that it will not return any value to the calling function which is main() function in this case.
User defined functions are written in 3 steps in C language.
- First of all we write a function prototype for the function before main() function normally.
- We call the user defined function within main() function by passing parameters if necessary. We will take care of any value returned by the called function, if any.
- We will write a function definition for the user defined function. The function definition will consists of all the statements of the function to perform the task.
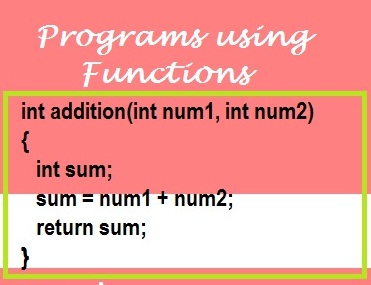
Source code for program add two numbers using a function that does not return a value in C programming is as follows:
/* Write a C program to add two numbers using function */ void addition(int a, int b); /*function prototype or declaration*/ #include<stdio.h> int main() { int num1,num2; /* Enter two numbers in main() */ printf("Enter two numbers for addition="); scanf("%d%d", &num1,&num2); /* call the function by sending two numbers */ addition(num1, num2); return 0; } /* write down function definition */ void addition(int a, int b) { int result; result = a + b; printf("\n Addition =%d", result); }
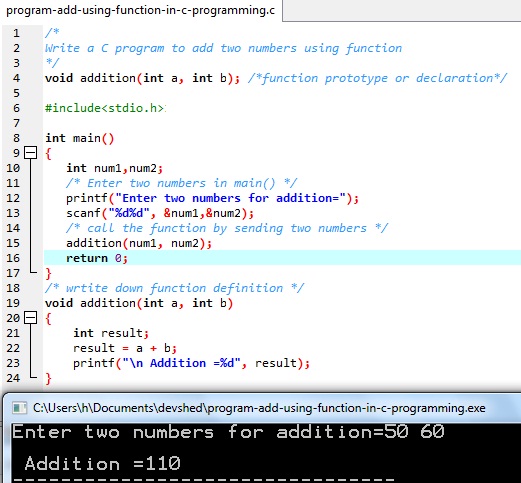
Program to add two numbers using function in C programming
Now here is the source code for a C program using a function add() that returns an integer value:
/* Write a C program to add two numbers using a user defined function */ int addition(int a, int b); /*function prototype or declaration*/ #include<stdio.h> int main() { int num1,num2, result; /* Enter two numbers in main() */ printf("Enter two numbers for addition="); scanf("%d%d", &num1,&num2); /* call the function by sending two numbers */ result = addition(num1, num2); printf("\n Addition =%d", result); return 0; } /* wrtite down function definition */ int addition(int a, int b) { int sum; sum = a + b; return sum; }