“Print star diamond pattern” is a star pattern program in C language to give the required output. This program uses for loop concept in C programming language and prints the diamond of stars according to given input about number of rows.
This C program uses the following set of statements:
- Pre processor directives to include header files
- printf() function is usd to show output stars
- scanf() function is used to enter number of rows by user at run time.
- It uses for loop according to the conditions of the program.
- This program uses a lot of loops and related counter variables.
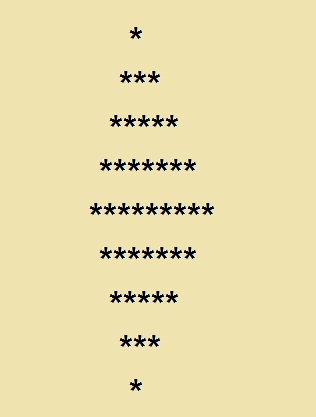
“Print star diamond pattern” is a star pattern program in C language to give the required output.
/* Write a C program to print star diamond pattern as shown below * *** ***** ******* ********* ******* ***** *** * */ #include<stdio.h> int main() { int n, i, j, space; printf("Enter the number of rows for diamond pattern: "); scanf("%d",&n); space = n - 1; for (j = 1; j <= n; j++) { for (i = 1; i <= space; i++) { printf(" "); } space--; for (i = 1; i <= 2 * j - 1; i++) { printf("*"); } printf("\n"); } space = 1; for (j = 1; j <= n - 1; j++) { for (i = 1; i <= space; i++) { printf(" "); } space++; for (i = 1; i <= 2 * (n - j) - 1; i++) { printf("*"); } printf("\n"); } }
Here is an image to show the sample run and output of diamond of stars pattern program in C language.
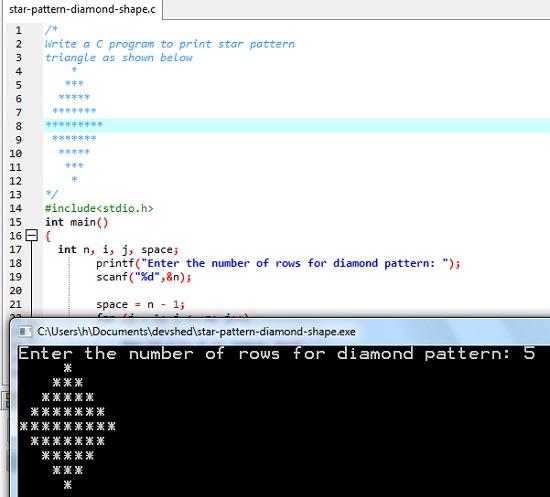
Here is an image to show the sample run and output of diamond of stars pattern program in C language.