“Array input output C program” is a C language program to input 10 integer numbers in a 10 element array. This program will show the entered elements on screen, too.
Array is a data structure which represents the collection of items of same type. For example, array of 10 integers may be defined as:
int num[10];
Where int is the data type, num is the name of array. Whereas 10 represents size of array. We can use an element with the help of index. For example to display first element , we will write
printf(“%d”, num[0]);
num[0] represents first element of array, similarly 9 will represent the index of last element of array.
As in C language array index is zero based, therefore a 10 elements array will use indices from 0 to 9 for 10 elements. The first element of array has index 0 and the last item of array has index 9.
For input and output from array, we use “for loop” for ease of use and efficiency of program.
/* Write a C Program to input ten numbers in array of ten integers. Display all values from array */ #include<stdio.h> int main() { int numArray[10], i; //input in array using for loop for(i=0; i<10; i++) { printf("Enter Element No:%d=",i+1); scanf("%d", &numArray[i]); } // show array elements using for loop printf("The elements of array are:\n"); for(i=0;i<10;i++) printf("%d\n", numArray[i]); return 0; }
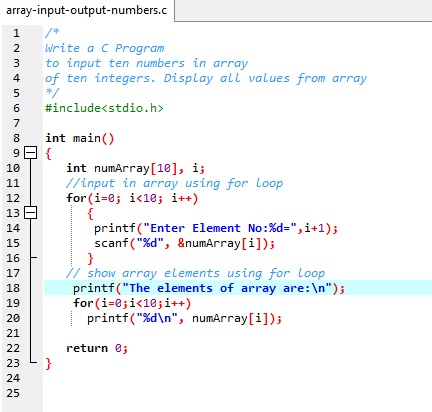
Output of the one dimensional array in C program
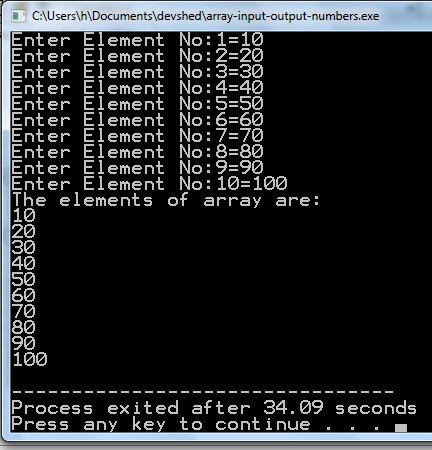
Array input output program in C language
Pingback: Input Output of Elements in Float Array - EasyCodeBook.com