Q: Write a C program to Search a Record in Binary File in C Programming.
What is the input of Record Search C Program?
This C program will input a field to start a search with. For example, in a student binary file, we will input roll number of the student to start search in c file.
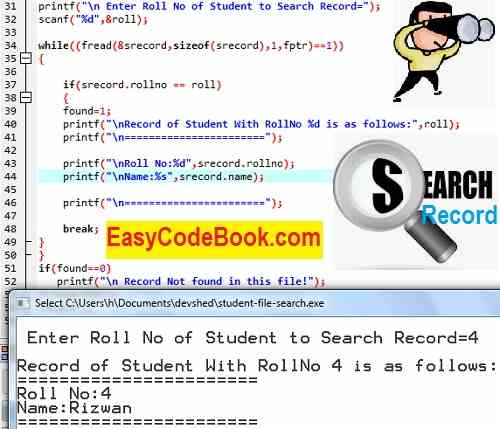
How To Search A Record In Binary File?
When we have a given roll number to start search with, we will open the binary file in read mode:
/*open binary file in read mode*/
fptr=fopen(“d://sfile.dat”,”rb”);
If the file has been opened successfully, we will use a while loop to read file one record at a time until EOF is reached.
First of all we use fread() function to read first record. The roll number of currently read record is matched with given roll no. if both are matched, we display record.
Similarly, the program will read all recordsĀ one by one and compare with given roll no. If no such record is in the binary file, we will display “Record Not Found Message”.
The source code of Record Search Program in Binary File using C Programming is:
/* Write a C program to input a Roll No then search the record of this student in a binary file */ #include<stdio.h> #include<conio.h> #include<stdlib.h> struct student { int rollno; char name[30]; }srecord; int main() { FILE *fptr; int found=0, roll; /*open binary file in read mode*/ fptr=fopen("d://sfile.dat","rb"); if(fptr==NULL) { printf("File could not open"); exit(0); } printf("\n Enter Roll No of Student to Search Record="); scanf("%d",&roll); while((fread(&srecord,sizeof(srecord),1,fptr)==1)) { if(srecord.rollno == roll) { found=1; printf("\nRecord of Student With RollNo %d is as follows:",roll); printf("\n======================="); printf("\nRoll No:%d",srecord.rollno); printf("\nName:%s",srecord.name); printf("\n======================="); break; } } if(found==0) printf("\n Record Not found in this file!"); fclose(fptr); return 0; }