Task: Steps to Get Keyboard Input in Basic Java Program
The Input For Java Console Applications ( Basic Java Programs )
Java Console Applications are the basic programs to start learning Java Programming Language. The new Java programmers wish to write general programs with run time input from the user. For example, suppose we wish to write a program to input a number and display its square. Therefore, on execution of this Java program, it will show a message to user for keyboard input. Hence, the user will enter a number and press Enter key. Here is a sample run of the program.
Enter a number to calculate square=
Now let us assume that the user will type 10 from the keyboard and press Enter key. The Java program will show the following output:
Enter a number to calculate square=10
Square = 100BUILD SUCCESSFUL (total time: 10 seconds)
Simple and Short Steps:
Step1: import Scanner class
import java.util.Scanner;
Step 2:Create an object let "keyboard" of Scanner class
by passing it System.in (standard system input device)
Scanner keyboard = new Scanner(System.in);
Step 3://Step 3
Show message to enter data
System.out.print("Enter a number to calculate square=");
Step 4:
call nextInt() method to initiate integer input
num = keyboard.nextInt();
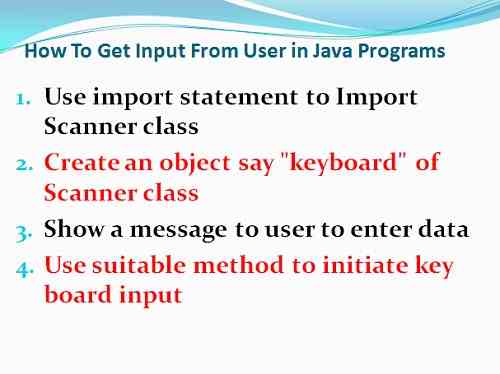
How to get user input from keyboard in Java Programs
How To Get Keyboard Input From User in Java Programs
We can write Java code to get input through keyboard from the user in the following four steps:
Use import statement to Import Scanner class
import java.util.Scanner;
Create an object say “keyboard” of Scanner class
Scanner keyboard = new Scanner(System.in);
Here keyboard is an object of Scanner class and we pass System.in to represent standard system input device that is keyboard.
Show a message to user to enter data
System.out.print("Enter a number to calculate square=");
Use suitable method to initiate key board input
For example, the method nextInt() is used to initiate integer number input from keyboard.
num = keyboard.nextInt();
Actually, when user types a number and presses Enter key from keyboard, say he types 10 and presses Enter. Consequently, 10 is stored in num variable on left side of assignment statement.
We can use num variable to calculate square of number as below:
square = num * num; And to show the result, we will write:
Syste.out.print("Square =" + square);
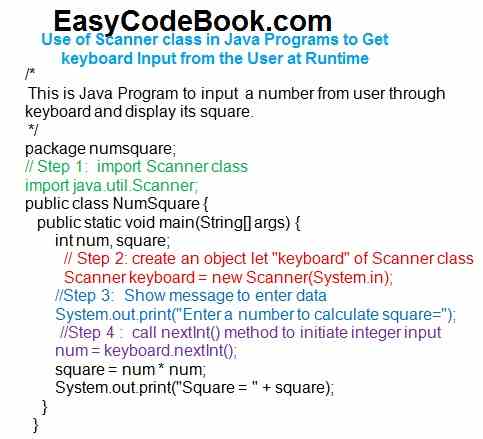
Use of Scanner class for keyboard input in Java programs
Getting Keyboard Input in Basic Java Program
Other useful methods in Scanner class for input of different type of data are as follows:
Method | Description |
---|---|
nextBoolean() |
Used to read a boolean value from the user |
nextByte() |
It is used to read a byte value from the user |
nextDouble() |
to read a double value from the user |
nextFloat() |
for reading a float value from the user |
nextInt() |
It is used to read a int value from the user |
nextLine() |
Use this method to read a String value from the user |
nextLong() |
We use it to read a long value from the user |
nextShort() |
It is used to read a short value from the user |
The complete source code of a Java Program explaining to Get Keyboard Input in Basic Java Program is here: Write a Java Program to input a number and display its square.
Pingback: Java Program To Check a Number is Prime or Composite » EasyCodeBook.com
Pingback: Java Program To Input a Number and Display Its Square | EasyCodeBook.com
Pingback: Write and Execute Java Addition Program | EasyCodeBook.com