Python if elif else Statement decides to select only one block of statements from many given block of statements. It selects a block on the basis of the condition related to that block. It means that if elif else statement has many conditions and corresponding blocks of statements. If a given condition is true, if elif else statement will execute the corresponding block.
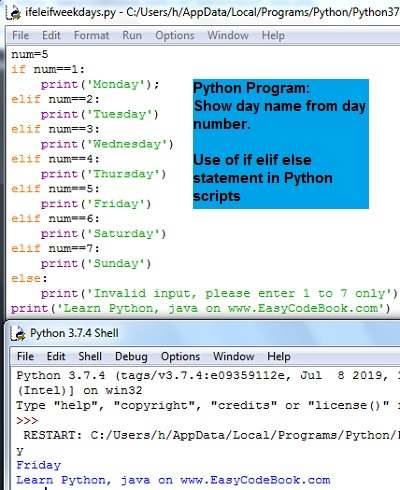
Syntax of Python if elif else Statement
The general form of if elif else statement is as follows:
if condition-if: if-block elif condition-1: block-1 elif condition-2 block-2 . . . elif condition-n: block-n else: else-block
Working:
First of all, it evaluates the condition-if. If it is true, it executes the if-block. It ignores rest of the if elif else statement.
If condition-if is false, the control will test first elif condition. If this condition is true, it will execute first elif block called block-1.
Similarly, if first elif condition is false, the control will move to second elif condition and evaluates it. If it is true then block-2 is executed, and so on.
If if-condition is false and all elif conditions are also false, then the control will execute else-block.
Example of if elif else Statement
Write a Python script to assign a number to a variable num. Use if elif else statement to show corresponding day. 1 is for Monday, 2 is for Tuesday and so on. if the number assigned is other than 1 to 7, show a message of ‘Invalid input’.
num=5 if num==1: print('Monday'); elif num==2: print('Tuesday') elif num==3: print('Wednesday') elif num==4: print('Thursday') elif num==5: print('Friday') elif num==6: print('Saturday') elif num==7: print('Sunday') else: print('Invalid input, please enter 1 to 7 only') print('Learn Python, java on www.EasyCodeBook.com')
The output of the above Python code will be as follows:
Friday
This is because the num variable has the value 5. Therefore,
elif num==5: condition will be true and it will execute corresponding block-5 which is a print statement / function: print(‘Friday’). Therefore, it displays the message ‘Friday’ on the screen.
Moreover, the last statement which is not a part of if elif else statement, will also display one more message as follows:
Learn Python, java on www.EasyCodeBook.com
if elif else Statement Example Program Find Grade
# Write a Python program # using if-elif-else to print # the grade based on # score input by the user # Perfect Python Programming Tutorials # Author : www.EasyCodebook.com (c) # Actual Program starts here # Python Program - Print Your Grade using if-elif-else score = int(input("Enter score: ")) if score >= 80: grade = 'A' elif score >= 70: grade = 'B' elif score >= 55: grade = 'C' elif score >= 50: grade = 'Pass' else: grade = 'Fail' print ("\nGrade is: " + grade)