Topic: Logical Operators in Python Language
Logical Operators in Python Language are used to create compound conditions. The compound conditions contain more than one conditions combined with logical operators. We use ‘and’ and ‘or’ logical operators to combine two or more conditions. Another logical operator ‘not’ is used to reverse the result of a condition.
a=5 b=10 if a==5 and b==10: print('Both conditions are True') The Result: Both conditions are True a=50 b=10 if a==5 and b==10: print('Both conditions are True') else: print('Any one or Both conditions are False') The Result: Any one or Both conditions are False gender='male' city = 'RYK' if gender == 'male' or city == 'RYK': print('Either candidate is male or from RYK city') else: print('Both conditions are wrong') The Result: Either candidate is male or from RYK city gender='female' city = 'SDK' if gender == 'male' or city == 'RYK': print('Either candidate is male or from RYK city') else: print('Both conditions are wrong') The Result: Both conditions are wrong
We use the Python comparison operators to create Python conditions like a > b or age>=18.
Purpose of Logical Operators in Python Language
We will use Python Logical operators if we want to combine two or more conditions or if we wish to reverse a condition.
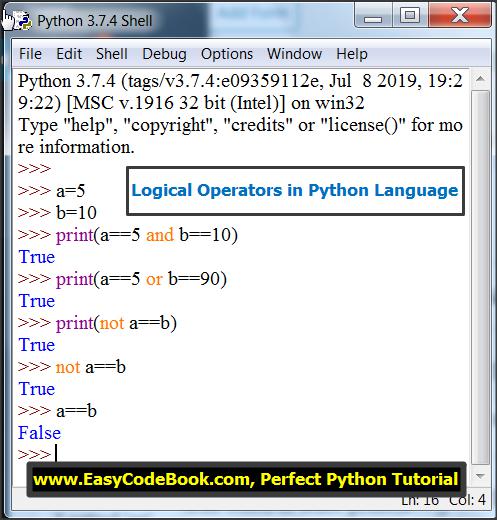
Logical Operators in Python Language
Types of Logical Operators in Python
There are three types of the logical operators in Python:
- Logical ‘and’ operator
- Logical ‘or’ operator
- Logical ‘not’ operator
LOGICAL OPERATOR | DEFINITION | EXAMPLE | VALUES | RESULT |
---|---|---|---|---|
Logical ‘and’ | ‘and’ will return true when the both conditions are true | print(a==5 and b==10) | a=5
b=10 |
True |
Logical ‘or’ | ‘or’ will return true when at least one of the given conditions is true | print(a==5 or b==90) | a=5
b=10 |
True |
Logical ‘not’ | ‘not’ will revert the result | print(not a==b) | a=5
b=10 |
True
{since a==b will give false and ‘not’ will convert the result to True } |
The Use of Logical ‘and’ Operator in Python
The ‘and’ logical operator is used in the progamming situations when we wish to have both conditions are true.
We say, ‘and’ logical operator will gives a True if both of the given condtions produce True result.
Example of ‘and’ Logical Operator
For example, if we wish to check for conditions like male and adult candidate for a job, we will write the two following conditions using logical ‘and’ operator and comparison operators:
if gender =’male’ and age>=18:
print(“A valid candidate: Male and Adult”)
else:
print(“Not a valid candiadate”)
Output: if we assume the following values for the variables:
age = 20
gender = ‘male’
Then the output will be:
A valid candidate: Male and Adult
On the other hand, if we assume the following values:
age = 17
gender = ‘male’
or
age = 20
gender = ‘female’
Then the output will be
Not a valid candiadate
The Truth Table for Logical ‘and’ Operator
A | B | A and B |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
The Use of Logical ‘or’ Operator in Python
The Logical ‘or’ operator is used in the progamming situations when we wish to have any one condition is true. That is, ‘or’ operator will give a True if any one of the two given conditions is True.
We say, ‘or’ logical operator will gives a True if any one of the both of the given condtions produce True result.
Example of ‘or’ Logical Operator
For example, if we wish to check for conditions like a valid candidate is either with an overall first division result of if he / she has experience of 5 or more years. We will write the two following conditions using logical ‘or’ operator and comparison operators:
if division = ‘first’ or experience >=5:
print(“A valid candidate”)
else:
print(“Not a valid candiadate”)
Output: if we assume the following values for the variables:
division =’first’
experience = 6
Then the output will be:
A valid candidate: Male and Adult
On the other hand, if we assume the following values:
age = 17
gender = ‘male’
or
age = 20
gender = ‘female’
Then the output will be
Not a valid candiadate
The Truth Table for Logical ‘or’ Operator
A | B | A or B |
---|---|---|
True | True | True |
True | False | True |
False | True | True |
False | False | False |
The Use of ‘not’ Logical Operator in Python
The logical operator ‘not’ is used to reverse the result of a given condition.
Example of ‘not’ Logical Operator in Python Language
a==50
print(a==50)
Result: True
print(not a==50)
Result: False [not since result of a==50 is True so after reversing the result is False]
The Truth Table for Logical ‘not’ Operator
A | not A |
---|---|
True | False |
False | True |