“C Program to check for Prime or Composite Number” is a C language program to input a number and check whether it is a prime number or composite number.
1 is Prime or Composite
The input should be a positive integer greater than one. Since we know that by definition of prime number, 1 is neither prime nor composite. A prime number has only two divisors whereas 1 has only one divisor.
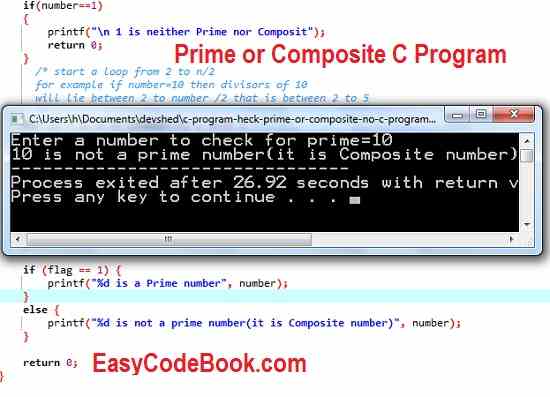
The Logic Behind Prime or Composite Checker C Program
Suppose we have to check a number =10 for prime. we know that a number is prime if it is divisible by 1 and itself. It means it has only two divisors. We know that any number will be divisible by 1 and number itself. So 10 is divisible by 1 and 10.
We also know that maximum divisor of a number(except itself) can be up to half of that number. It means that the divisors of 10 will lie between 2 to 5 (ignoring 1 and 10, we already know).
We will use a loop for example a for loop to get numbers from 2 to n/2 that is from 2 to 5. Each number will divide 10. As soon as first number 2 will divide 10, it is clear that 10 has now more than two divisors so we assign zero to flag and break the loop.
Source code for prime or Composite checking program is as follows:
/* Write a C program to check whether a given number is prime or composite */ #include <stdio.h> int main() { int number, i, flag = 1; /* by flag =1 we assume that given number is prime */ /* display a message to user for input */ printf("Enter a number to check for prime="); /* get a number from user at run time */ scanf("%d", &number); if(number==1) { printf("\n 1 is neither Prime nor Composit"); return 0; } /* start a loop from 2 to n/2 for example if number=10 then divisors of 10 will lie between 2 to number /2 that is between 2 to 5 */ for (i = 2; i <= (number/2) ; i++) { /* If there is a divisor between 2 and half of number(n/2) , we assign zero to flag, indicating 'not prime' */ if (number % i == 0) { flag = 0; break; } } if (flag == 1) { printf("%d is a Prime number", number); } else { printf("%d is not a prime number(it is Composite number)", number); } return 0; } /*Output: Enter a number: 13 13 is a Prime number */
You may also like to read this C program:
C Program To Check a number for prime or Composite using c user defined functions