“C Program Using Ternary Operator To Check Even Odd”
This is a C program that uses conditional operator in C programming language to check whether a number is even or odd.
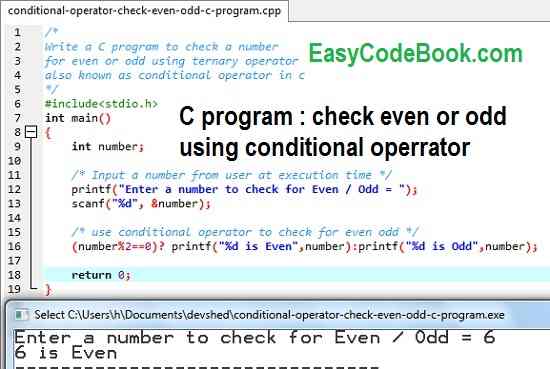
Ternary operator is called conditional operator in C programming language. The syntax of ternary operator is as follows:
(condition)? true-case-statement : false-case-statement;
Example of using ternary operator in c programming language is:
x=100;
ans=(x>10)? 1:0;
It is equivalent to if else statement:
if (x>10)
ans=1;
else
ans=0;
Now since x>10 condition is true so 1 will be assigned to ans variable. If the condition is wrong then zero will be assigned to variable ans.
Source code of checking even odd program using conditional or ternary operator is as follows:
/* Write a C program to check a number for even or odd using ternary operator also known as conditional operator in c */ #include<stdio.h> int main() { int number; /* Input a number from user at execution time */ printf("Enter a number to check for Even / Odd = "); scanf("%d", &number); /* use conditional operator to check for even odd */ (number%2==0)? printf("%d is Even",number):printf("%d is Odd",number); return 0; }
Pingback: if else statement even odd c program - EasyCodeBook.com