Python Program Print Stars Rectangle – To print a rectangle of stars in Python, you can use nested loops similar to the square example, but you’ll need to specify the number of rows and columns for the rectangle.
Source code
# Get the number of rows and columns from the user rows = int(input("Enter the number of rows: ")) columns = int(input("Enter the number of columns: ")) # Nested loop to print the rectangle of stars for i in range(rows): for j in range(columns): print("*", end=" ") print()
Here’s a simple program to print a rectangle of stars:
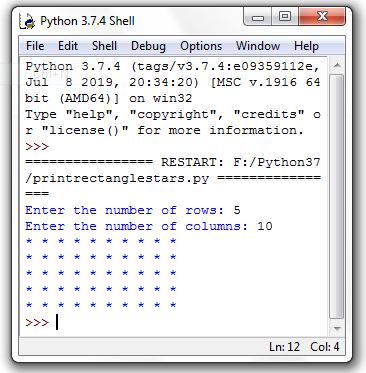
Output
Enter the number of rows: 5
Enter the number of columns: 10
* * * * * * * * * *
* * * * * * * * * *
* * * * * * * * * *
* * * * * * * * * *
* * * * * * * * * *In this program:
Enter the number of rows: 5
Enter the number of columns: 10
* * * * * * * * * *
* * * * * * * * * *
* * * * * * * * * *
* * * * * * * * * *
* * * * * * * * * *In this program:
- We ask the user to enter the number of rows and columns for the rectangle.
- We use two nested
for
loops to iterate through the rows and columns of the rectangle. - In the inner loop, we print a star followed by a space to separate the stars.
- After each row is printed (the inner loop completes), we use
print()
to move to the next line, so the stars are printed in rows.
You can adjust the rows
and columns
variables to control the dimensions of the rectangle. For example, if the user enters 3 for rows and 5 for columns, the program will print a rectangle of stars with 3 rows and 5 columns.