Python Program Print Hollow Diamond Star Pattern-
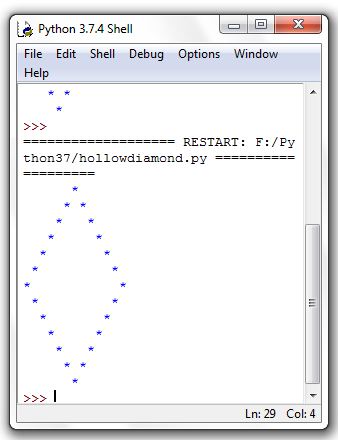
Python Program Print Hollow Diamond Star Pattern
# Number of rows row = 7 # this code will print upper part of hollow diamond pattern for i in range(1, row+1): for j in range(1,row-i+1): print(" ", end="") for j in range(1, 2*i): if j==1 or j==2*i-1: print("*", end="") else: print(" ", end="") print() # now print Lower part of hollow diamond shape for i in range(row-1,0, -1): for j in range(1,row-i+1): print(" ", end="") for j in range(1, 2*i): if j==1 or j==2*i-1: print("*", end="") else: print(" ", end="") print()
This code is used to print a hollow diamond shape made of asterisks (*) and spaces. Here’s an explanation of the code:
row = 7
: This line defines the variablerow
and sets it to 7, which determines the number of rows in the diamond. You can change this value to create a diamond of a different size.- The code uses two loops: one for the upper part of the diamond and another for the lower part.
for i in range(1, row+1)
: This loop iterates from 1 to the specified number of rows (inclusive) for the upper part of the diamond.for j in range(1, row-i+1)
: This loop prints leading spaces before the asterisks in each row. It calculates the number of spaces based on the current rowi
to create the shape of the diamond.for j in range(1, 2*i)
: This loop prints the asterisks and spaces within each row. It iterates from 1 to2*i
. Ifj
is equal to 1 or2*i-1
, it prints an asterisk, and for all other values ofj
, it prints a space to create the hollow effect.- The
if j==1 or j==2*i-1
condition is used to print an asterisk at the beginning and end of each row while leaving spaces in the middle. print()
: This is used to move to the next line to start the next row.- After the upper part of the diamond is printed, there’s another loop for the lower part of the diamond:
for i in range(row-1, 0, -1)
: This loop iterates fromrow-1
down to 1 for the lower part of the diamond.- The structure of this loop is similar to the upper part, printing leading spaces, asterisks, and spaces to create the hollow effect.
This code results in a diamond shape made of asterisks with a hollow center. The center is left empty with spaces. You can adjust the row
variable to change the size of the diamond.