Basic PyQt Tutorial With Common Widgets –
Creating a basic PyQt application involves creating a graphical user interface (GUI) using the PyQt library, which is a set of Python bindings for the Qt application framework.
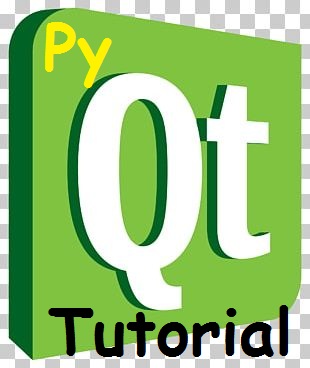
Basic PyQt Tutorial With Common Widgets
In this tutorial, we’ll walk through the steps to create a simple PyQt application.
Step 1: Install PyQt
Before you start, make sure you have PyQt installed. You can install it using pip:
pip install PyQt5
Step 2: Create a Basic PyQt Application
Create a Python script (e.g., basic_pyqt_app.py
) and add the following code to create a minimal PyQt application with a window:
import sys from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton # Create a QApplication app = QApplication(sys.argv) # Create a QMainWindow window = QMainWindow() window.setWindowTitle("Basic PyQt Application") window.setGeometry(100, 100, 400, 200) # (x, y, width, height) # Create a QLabel label = QLabel("Hello, PyQt!", parent=window) label.setGeometry(150, 50, 200, 30) # (x, y, width, height) # Create a QPushButton button = QPushButton("Click Me!", parent=window) button.setGeometry(150, 100, 100, 30) # (x, y, width, height) # Define a function to execute when the button is clicked def on_button_click(): label.setText("Button Clicked!") # Connect the button's click event to the function button.clicked.connect(on_button_click) # Show the main window window.show() # Run the application's event loop sys.exit(app.exec_())
In this code:
- We import the necessary PyQt classes and create an instance of
QApplication
to manage the application’s main settings. - We create a
QMainWindow
instance, set its title and geometry, and create a label and a button. - We define a function
on_button_click()
that changes the label text when the button is clicked. - We connect the button’s
clicked
signal to theon_button_click()
function. - Finally, we start the application’s event loop with
app.exec_()
.
Step 3: Run the Application
To run the application, execute the Python script from the command line:
python basic_pyqt_app.py
You should see a window with a label and a button. Clicking the button should change the label text.
Introduction To PyQt Widgets with Properties and Methods
PyQt is a Python library for creating desktop applications with graphical user interfaces (GUIs). It provides a wide range of widgets, each of which represents a specific GUI element such as buttons, labels, text boxes, and more. These widgets come with various properties and methods that allow you to customize their appearance and behavior. In this introduction, we’ll explore some commonly used PyQt widgets, their properties, and methods.
1. QLabel:
QLabel
is used for displaying text or images on the GUI.
Properties:
text
: Gets or sets the text displayed on the label.alignment
: Sets the alignment of the text within the label (e.g., left, center, right).font
: Sets the font used for the text.
Methods:
setText(text)
: Sets the text displayed on the label.setAlignment(alignment)
: Sets the text alignment.
2. QPushButton:
QPushButton
is a clickable button that can trigger actions when clicked.
Properties:
text
: Gets or sets the text displayed on the button.enabled
: Enables or disables the button.clicked
: Signal emitted when the button is clicked.
Methods:
setText(text)
: Sets the text displayed on the button.setEnabled(enabled)
: Enables or disables the button.click()
: Programmatically simulates a button click.
3. QLineEdit:
QLineEdit
is a single-line text input field.
Properties:
text
: Gets or sets the text entered in the line edit.placeholderText
: Sets a placeholder text to provide context for the input.
Methods:
setText(text)
: Sets the text in the line edit.text()
: Retrieves the text from the line edit.
4. QMainWindow:
QMainWindow
is a top-level application window.
Properties:
windowTitle
: Gets or sets the title of the main window.menuBar
: Sets the menu bar for the main window.
Methods:
setWindowTitle(title)
: Sets the window title.setMenuBar(menuBar)
: Sets the menu bar.
5. QMessageBox:
QMessageBox
is used to display informative or error messages to the user.
Properties:
icon
: Sets the message box’s icon (e.g., information, warning, critical).text
: Sets the main text of the message box.standardButtons
: Sets the standard buttons (e.g., Ok, Cancel) displayed in the message box.
Methods:
setIcon(icon)
: Sets the message box’s icon.setText(text)
: Sets the main text.setStandardButtons(buttons)
: Sets the standard buttons.
introduction to CheckBox, RadioButton, ListBox, and ComboBox widgets in PyQt, along with their common properties and methods:
1. CheckBox:
A QCheckBox
is a widget that represents a binary choice, allowing users to select or deselect an option.
Common Properties:
text
: Gets or sets the label text displayed next to the checkbox.isChecked
: Gets or sets whether the checkbox is checked (True) or unchecked (False).
Common Methods:
setText(text)
: Sets the label text for the checkbox.isChecked()
: Returns True if the checkbox is checked; otherwise, returns False.
2. RadioButton:
QRadioButton
is used to create exclusive choices where only one option can be selected from a group of radio buttons.
Common Properties:
text
: Gets or sets the label text displayed next to the radio button.isChecked
: Gets or sets whether the radio button is checked (True) or unchecked (False).
Common Methods:
setText(text)
: Sets the label text for the radio button.isChecked()
: Returns True if the radio button is checked; otherwise, returns False.
3. ListBox:
QListWidget
is used to display a list of items from which users can select one or more items.
Common Properties:
addItem(item)
: Adds an item to the list.currentItem
: Gets or sets the currently selected item.selectedItems
: Returns a list of selected items.
Common Methods:
addItem(item)
: Adds an item to the list.takeItem(row)
: Removes and returns an item from the specified row.clear()
: Removes all items from the list.setCurrentItem(item)
: Sets the currently selected item.setSelectionMode(mode)
: Sets the selection mode (e.g., SingleSelection, MultiSelection).
4. ComboBox:
A QComboBox
is used to create a dropdown list of items from which users can select one option.
Common Properties:
addItem(item)
: Adds an item to the combo box.currentText
: Gets or sets the text of the currently selected item.currentIndex
: Gets or sets the index of the currently selected item.count
: Gets the number of items in the combo box.
Common Methods:
addItem(item)
: Adds an item to the combo box.clear()
: Removes all items from the combo box.setCurrentText(text)
: Sets the currently selected item based on its text.setCurrentIndex(index)
: Sets the currently selected item based on its index.count()
: Returns the number of items in the combo box.
These widgets are fundamental in creating interactive user interfaces with PyQt. You can use them to capture user input, provide options for selection, and display lists of items. Depending on your application’s requirements, you can customize their properties and utilize their methods to enhance user interaction and functionality.
Sample PyQt Application Write a PyQt application to Display a List Box With 1 to 10 Numbers. When User clicks on a number in List box. Factorial of the number will display on a Label.
import sys import math from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QListWidget, QVBoxLayout, QWidget class FactorialCalculator(QMainWindow): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setWindowTitle("Factorial Calculator") self.setGeometry(100, 100, 300, 200) # Create a central widget central_widget = QWidget(self) self.setCentralWidget(central_widget) # Create a vertical layout for the central widget layout = QVBoxLayout() # Create a label to display the factorial self.label_result = QLabel("Factorial: ", self) layout.addWidget(self.label_result) # Create a list box to display numbers self.list_box = QListWidget(self) for i in range(1, 11): self.list_box.addItem(str(i)) self.list_box.clicked.connect(self.calculate_factorial) layout.addWidget(self.list_box) central_widget.setLayout(layout) def calculate_factorial(self): selected_item = self.list_box.currentItem() if selected_item: number = int(selected_item.text()) factorial = math.factorial(number) self.label_result.setText(f"Factorial: {factorial}") if __name__ == "__main__": app = QApplication(sys.argv) calculator = FactorialCalculator() calculator.show() sys.exit(app.exec_())