“Unleashing the Magic of Operator Overloading: When Objects Dance with Operators!”
Operator Overloading
Operator overloading in C++ enables you to redefine or extend the behavior of existing operators for user-defined data types or objects.
For example, in C++, the + operator is used to perform addition for built-in data types like integers and floating-point numbers. It cannot add two objects. With operator overloading, you can redefine the + operator to add two objects of your own class. This will allow you to add two objects in a meaningful way.
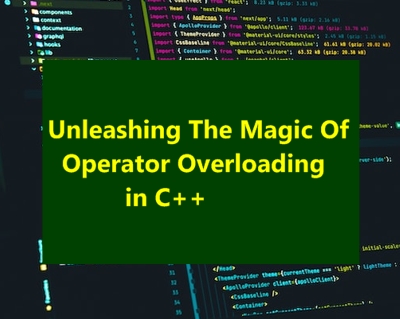
Unleashing the Magic of Operator Overloading
Breaking Boundaries: Why Operator Overloading is the Game Changer You Need!
The need for operator overloading arises from several reasons:
Simplified Syntax: Operator overloading allows you to use familiar operators to perform operations on custom objects. This makes the code more concise and easier to read.
Enhanced Readability: Operator overloading can lead to more expressive and readable code
Code Reusability: Operator overloading promotes code reusability by allowing you to use the same operator for different classes.
Consistency with Built-in Types: Operator overloading enables you to maintain consistency with the behavior of built-in types.
Unlock the Secrets of Operator Overloading: Explore Captivating C++ Source Code!
#include<iostream> using namespace std; class Box { private: double length; double width; double height; public: // Constructor Box(double len, double wid, double ht) : length(len), width(wid), height(ht) {} // Overloaded + operator to add two Box objects Box operator+(const Box& other) { double sumLength = length + other.length; double sumWidth = width + other.width; double sumHeight = height + other.height; return Box(sumLength, sumWidth, sumHeight); } // Member function to set the dimensions of the box void setBox(double len, double wid, double ht) { length = len; width = wid; height = ht; } // Member function to display the dimensions of the box void showBox() { cout << "Box Dimensions: " << length << " x " << width << " x " << height << endl; } }; int main() { // Create two Box objects Box box1(3.0, 4.0, 5.0); Box box2(2.0, 3.0, 4.0); // Set the dimensions of box1 and box2 box1.setBox(3.0, 4.0, 5.0); box2.setBox(2.0, 3.0, 4.0); // Add box1 and box2 to create a third box (box3) Box box3 = box1 + box2; // Display the dimensions of all three boxes cout << "Box 1: "; box1.showBox(); cout << "Box 2: "; box2.showBox(); cout << "Box 3 (Result of Addition): "; box3.showBox(); return 0; }
Output of C++ Program Operator Overloading
Box 1: Box Dimensions: 3 x 4 x 5
Box 2: Box Dimensions: 2 x 3 x 4
Box 3 (Result of Addition): Box Dimensions: 5 x 7 x 9
Explanation of Output Achieved
We first set the dimensions of box1 using box1.setBox(3.0, 4.0, 5.0) and the dimensions of box2 using box2.setBox(2.0, 3.0, 4.0).
Then, we use the overloaded + operator to add box1 and box2, which creates a new Box object box3 with dimensions (3 + 2, 4 + 3, 5 + 4) = (5, 7, 9).
Finally, we display the dimensions of all three boxes using the showBox() method, resulting in the output as shown above.
Brief Explanation of Source Code
- The Box class has private member variables length, width, and height to represent the dimensions of the box.
- The constructor of the Box class is used to initialize the dimensions of the box when an object is created.
- The + operator is overloaded as a member function of the Box class. It takes another Box object as a parameter and returns a new Box object with the sum of their dimensions.
- The setBox() member function allows us to set the dimensions of the box.
- The showBox() member function displays the dimensions of the box.
- In the main() function, we create two Box objects (box1 and box2) and set their dimensions using the setBox() method.
We then add box1 and box2 together using the overloaded + operator, which results in a new Box object (box3) with the sum of their dimensions. - Finally, we display the dimensions of all three boxes using the showBox() method.