C Program Remove Blank Spaces from a given string entered by the user at run time.
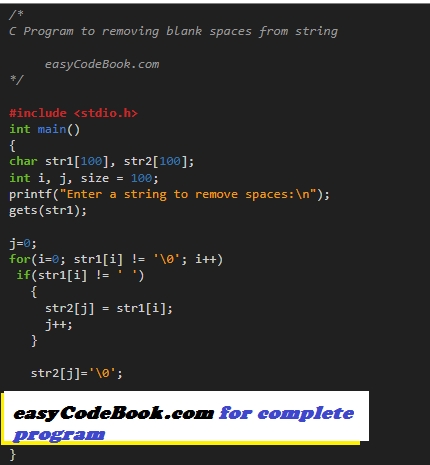
Code
/* C Program to removing blank spaces from string easyCodeBook.com */ #include <stdio.h> int main() { char str1[100], str2[100]; int i, j, size = 100; printf("Enter a string to remove spaces:\n"); gets(str1); j=0; for(i=0; str1[i] != '\0'; i++) if(str1[i] != ' ') { str2[j] = str1[i]; j++; } str2[j]='\0'; printf("String with out blank spaces is: \n"); puts(str2); return 0; }
Output:
Enter a string to remove spaces: Hello World String with out blank spaces is: HelloWorld
Explanation of Logic
The given C program removes blank spaces from a string. It reads a string from the user, iterates through each character, and copies non-space characters to a new string. Finally, it prints the modified string without blank spaces.
gets(str1);
- The program starts with a multi-line comment providing a description of the program.
- The code includes the standard input/output library
stdio.h
. - The
main
function is defined as the entry point of the program. - Inside the
main
function, several variables are declared:char str1[100]
andchar str2[100]
are arrays of characters.str1
is the original string, andstr2
will store the modified string without blank spaces.int i
andint j
are integer variables used as indices for iterating through the characters of the strings.int size
is an integer variable initialized to 100, which represents the maximum size of the strings.
- The
printf
function is used to display a prompt message asking the user to enter a string to remove spaces. - The
gets
function is used to read a line of input from the user and store it in thestr1
array.
- The code enters a
for
loop that iterates through each character of thestr1
string until the null character'\0'
is encountered, indicating the end of the string. - Inside the loop, an
if
condition checks if the current characterstr1[i]
is not a space' '
. If the condition is true, the character is copied to thestr2
string at indexj
, andj
is incremented. - After each iteration, the indices
i
andj
are incremented to move to the next character in thestr1
string and the next available position in thestr2
string, respectively.
- After the loop finishes, the null character
'\0'
is explicitly added to thestr2
string at indexj
to mark the end of the string. - The
printf
function is used to display a message indicating that the string without blank spaces is about to be printed. - The
puts
function is used to print the modified stringstr2
, which contains the original string without any blank spaces. - The
return 0;
statement indicates the end of themain
function, and it signifies that the program execution was successful.