Topic: Python Set Label Text on Button Click tkinter GUI Program
# Write a Python GUI program # using tkinter module # to set text "Easy Code Book" in Label # on button click. import tkinter as tk def main(): window= tk.Tk() window.title("Show Label and Button Widgets") window.geometry("400x200") # create a label with some text label1 = tk.Label(window, text="") # place this label in window at position x,y label1.place(x=130,y=50) txt = "Easy Code Book" def btn1_click(): label1.configure(text = txt) # create a button with text Button 1 btn1 = tk.Button(window, text="Click Me", command=btn1_click) # place this button in window at position x,y btn1.place(x=150,y=100) window.mainloop() main()
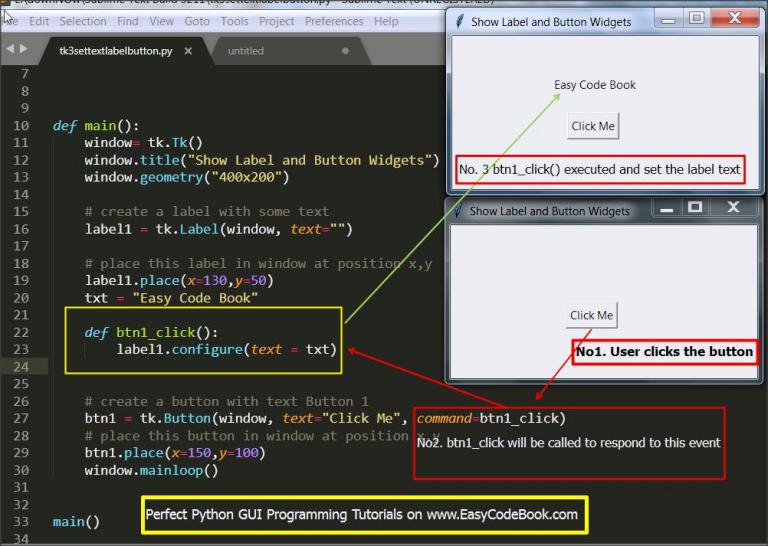
Explanation of Code in the Image
- Comments
- Comments
- Comments
- Comments
- Blank
- Imports tkinter module, use tk as an alias. Because tk is short and easy to use in code.
- Header of main() function.
- Indented body of main() function . Create main window or Root window of the application.
- Set title of the main window.
- Set size of the main window.
- Comments
- Create a label with empty text.
- Comments
- Place label in the main window at x,y location.
- Define and set a txt variable to “Easy Code Book” string literal.
- Define btn1_click() function to handle the button click event.
- Set the text of label to txt variable.
- Comments
- Create a button and bind it to btn1_click event handler by setting command option.
- Comments
- Place button in the root window at x,y position.
- When this line of code will execute, it will start the mainloop that is event loop. So that the output root window is shown to the user. It will wait for any actions called events performed by the user and the code attached, if any, will respond to those events. For example, if we click on close button, the window will be closed.
- Call to main() function. Now the execution of the program will start and the root window will be displayed on the screen.
Pingback: Multiplication Table Python GUI Program | EasyCodeBook.com