Topic: Python Sentinel Controlled Loop Example Program
What is a Sentinel Controlled Loop inPython?
A Sentinel Controlled Loop is a loop whose execution depends upon a special value. This sentinal controlled loop is terminated if a special value called sentinel value is encountered.
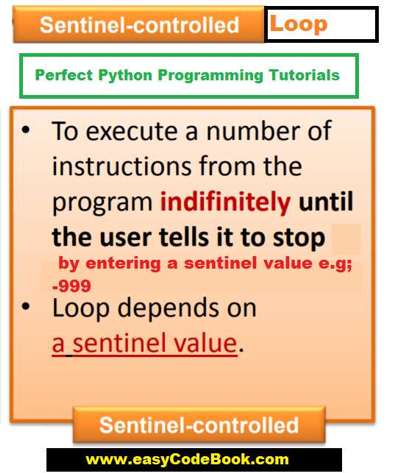
Python Sentinel Controlled Loop Example Program
The sentinel controlled loops are conditional loops. For example we can use a while loop as a sentinl controlled as follows:
Exapmple Of a Sentinel Controlled Loop Situation
Suppose that we have to take n nubers from the user at run time and to show their sum. We do not know the value of n in advance. It depends on the will of the user if he / she enters 10 numbers, 20 numbers or 31 numbers.
Solution to this Problem by Sentinel Controlled Loop
Suppose we choose -999 as sentinel value. Therefore we will use a while loop to take a number from user repeatedly and sum it as long as it is not -999. The loop will terminated if the user enters -999.
Here is the complete program to solve this problem using a while loop as sentinel controlled loop.
# An example Python program # to show the use of Sentinel controlled loops # The user will be allowed to enter numbers for adding # until he enter -999 to stop. # the program then will show the sum of all # numbers entered exxcept -999 sentinel value. # Author : www.EasyCodebook.com (c) sum = 0 # sum of numbers num = input( "Enter a number to add, (Enter -999 to STOP): " ) num = int(num) # convert string to an integer number while num != -999: # -999 is used as a sentinel value to stop sum = sum + num num = input( "Enter a number to add, (Enter -999 to STOP): " ) num = int( num ) print ("The Sum of all entered numbers is:", sum)
Output
Enter a number to add, (Enter -999 to STOP): 2 Enter a number to add, (Enter -999 to STOP): 4 Enter a number to add, (Enter -999 to STOP): 5 Enter a number to add, (Enter -999 to STOP): 9 Enter a number to add, (Enter -999 to STOP): -999 The Sum of all entered numbers is: 20
How this sentinel controlled loop works?
First of all the user input a number 2 before entering in loop.
The loop condition verifies num=2 is not equal to -999, so the control will enter into loop body. Note that sum is initialized to 0 before loop.
The number 2 is added to sum, sum=2 now.
The program asks the user again, to enter number or -999 to stop . The user enters 4. The loop body ends. So loop condition is checked again. 4 is not equal to -999. The loop continues. 4 is added to sum. So sum is 6 now.
The user when asked, enters a 5. 5 is not equal to -999. So 5 isadded to sum. The sum is 11 now.
The user next time, enters a 9. 9 is not equal to -999. So 9 isadded to sum. The sum is 20 now.
At last the user enters -999 when asked to enter a number or -999 o stop.
Now -999!=-999 condition becomes false. Hence loop is terminated with sentinel value -999.
Topic: Python Sentinel Controlled Loop Example Program
You will also like to read:
The Python while loop Syntax, Flowchart, Working, with Real World Examples
Python for Statement Syntax & Examples
Use of range() function in for loop with Examples