Topic: Python 3 GUI Program Add Two Numbers
This Python 3 GUI program uses tkinter module to create 4 label widgets, two entry widgets and one button. The user will enter two numbers in the two entry widgets. The result of addition will be shown in result label when the button is clicked by the user.
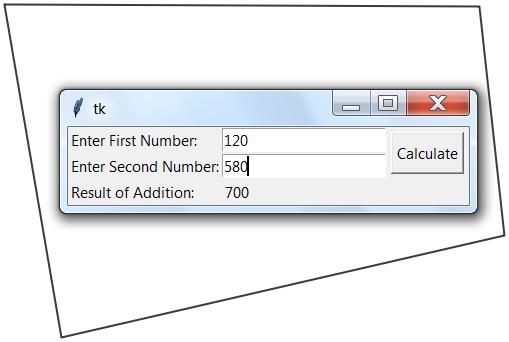
Python 3 GUI Program Add Two Numbers
# Python GUI program to # add two numbers # Using Labels, Entry and Button # widgets - Python 3 tkinter module from tkinter import * def add_numbers(): res=int(e1.get())+int(e2.get()) label_text.set(res) window = Tk() label_text=StringVar(); Label(window, text="Enter First Number:").grid(row=0, sticky=W) Label(window, text="Enter Second Number:").grid(row=1, sticky=W) Label(window, text="Result of Addition:").grid(row=3, sticky=W) result=Label(window, text="", textvariable=label_text).grid(row=3,column=1, sticky=W) e1 = Entry(window) e2 = Entry(window) e1.grid(row=0, column=1) e2.grid(row=1, column=1) b = Button(window, text="Calculate", command=add_numbers) b.grid(row=0, column=2,columnspan=2, rowspan=2,sticky=W+E+N+S, padx=5, pady=5) mainloop()
How this program works
# import statement for tkinter from tkinter import * # This is a user defined function to add two numbers # e1.get() will return text within text box (entry widget) # int() funcion will convert string to integer # both int numbers from two entry widgets are added and # result is assigned to res variable. # which is set into label_text string variable def add_numbers(): res=int(e1.get())+int(e2.get()) label_text.set(res) # get main window window = Tk() # bind the label widget to a StringVar instance, # and set or get the label text via that variable: label_text=StringVar(); # create 4 label widgets and place them in window using grid() Label(window, text="Enter First Number:").grid(row=0, sticky=W) Label(window, text="Enter Second Number:").grid(row=1, sticky=W) Label(window, text="Result of Addition:").grid(row=3, sticky=W) result=Label(window, text="", textvariable=label_text).grid(row=3,column=1, sticky=W) # create two entry widgets and place them in window # using grid() Grid Geometry Manager e1 = Entry(window) e2 = Entry(window) e1.grid(row=0, column=1) e2.grid(row=1, column=1) # create a button and place it in grid # Set command = add_numbers, so that when this button is clicked # add_numbers() function will be called. b = Button(window, text="Calculate", command=add_numbers) b.grid(row=0, column=2,columnspan=2, rowspan=2,sticky=W+E+N+S, padx=5, pady=5) # start mainloop, start GUI window.mainloop() You may also like to read PyQt GUI Version of this program
Pingback: Python GUI Area of Triangle | EasyCodeBook.com