C Program Sort N Strings in Ascending Order
/* C program to input n strings and sort them in ascending order alphabetically */ #include <stdio.h> #include <string.h> int main() { char str[30][40],temp[30]; int n,i,j; printf("\nString Sorting: Sorts N strings:\n"); printf("************************************\n"); printf("Input number of strings :"); scanf("%d",&n); getchar(); printf("Input string %d :\n",n); for(i=0;i<n;i++) gets(str[i]); /*Logic for sorting array of strings*/ for (i = 0; i < n; ++i) { for (j = i + 1; j < n; ++j) { if (strcmp(str[i], str[j]) > 0) { strcpy(temp, str[i]); strcpy(str[i], str[j]); strcpy(str[j], temp); } } } /* print the sorted array of strings */ printf("The strings in ascending order after sorting :\n"); for(i=0;i<n;i++) printf("%s\n",str[i]); return 0; }
Output
String Sorting: Sorts N strings:
************************************
Input number of strings :7
Input string 7 :
jameel jami
ahmad
zahid
umair
nadim
sajjad
babar
The strings in ascending order after sorting :
ahmad
babar
jameel jami
nadim
sajjad
umair
zahid
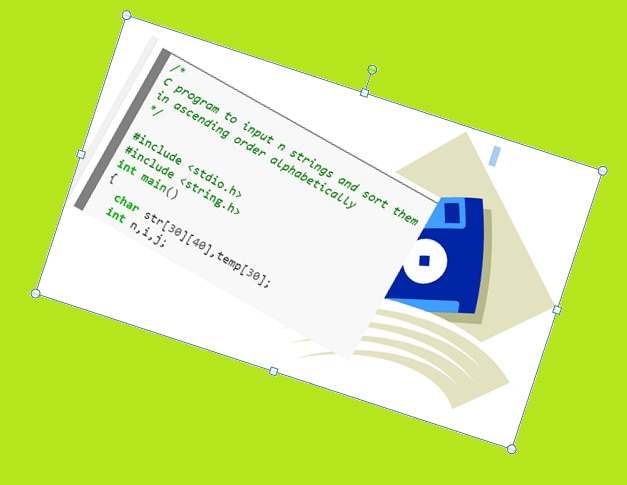
C Program Sort array of strings in ascending order
Sorting Array of N Strings in C
This C program will receive the size of array of strings from the user. The user will input N strings. This C program will sort these strings alphabetically and will show the strings in ascending order after sorting.
This program uses:
- gets(str) function for string input
- strcmp(str1,str2) function for comparing two strings
- strcpy(deststr,sourcestr) function to copy source string to deststring.
- Nested for loops for sorting strings in ascending order alphabetically / lexicographically
- getchar() function is used to absorb the Enter/Return key after entering N value. So that the first string may not take that Enter / Return key stroke with empty string as first string in the string array.
Therefore, this C program will perform string sorting in an efficient way using a simple sorting logic. The user will input how many number of strings to enter. In next step he / she will enter the N strings. The strings are sorted then in ascending order.