Python Program Factorial Three Versions – This Python tutorial explains three different source codes of the Python factorial program.
Write a program that asks the user for a number and prints out the factorial of that number:
- UsingĀ factorial() function of math module
- Using for loop
- Using while loop
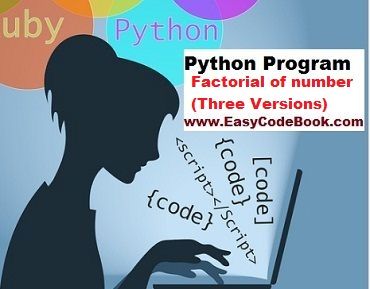
The Python has a math module. This math module contains a factorial() function that takes a positive integer as argument and returns the factorial of that number.
How to use Python factorial() function
We will use factorial() function after importing math module in our Python program.
Python Program Factorial Three Versions
The Source code of the Python factorial Program using factorial function
# Write a program that asks the user # to enter a number. # The program should display the factorial # of that number # Author: www.EasyCodeBook.com (c) from math import factorial num = int(input('Enter a number to find its factorial:')) fact = factorial(num) print('The Factorial of', num,'is:',fact)
The output is: Enter a number to find its factorial:4 The Factorial of 4 is: 24
Python Factorial Program using for loop
# Write a program that asks the user # to enter a number. # The program should display the factorial # of that number using for loop. # Author: www.EasyCodeBook.com (c) num = int(input('Enter a number to find its factorial:')) fact = 1 for i in range(1,num+1): fact = fact * i print('The Factorial of', num,'is:',fact)
The output is as follows: Enter a number to find its factorial:5 The Factorial of 5 is: 120
Python Factorial program using while loop
# Write a program that asks the user # to enter a number. # The program should display the factorial # of that number using while loop. # Author: www.EasyCodeBook.com (c) num = int(input('Enter a number to find its factorial:')) i = fact = 1 while i<=num: fact = fact * i i+=1 print('The Factorial of', num,'is:',fact)
The output of factrial program using while loop is: Enter a number to find its factorial:6 The Factorial of 6 is: 720