Task: Define Circle Class in Java with Radius and Area
How To Define Circle Class in Java
First of all, let’s consider the specification of a Circle class:
Write a Java Program to create a class Circle
with the following features:
Fields of Circle Class
1. A field radius of type double
2. a constant PI with value 3.14159
Constructors of Circle Class
1. A no arg constructor to set radius to 0.0
2. A parameterized constructor with one parameter
to assign a passed value to radius.
Methods of the Circle Class
Getters and Setters for Circle class:
1. setRadius() : A setter method
(also known as mutator method) for radius field
2. getRadius(): A setter method
( also known as accessor method for radis)
Other Methods of Circle Class
getArea(): calculates and returns area of circle
In addition, write a CircleTest class with the main() method.
The TestCircle class uses the Circle class to
create two objects: one with no arg constructor and
one with the parameterized constructor to initialize
the radius with 3.5. Call the methods of objects within
main() method of Circletest class.
How to Calculate Area of Circle?
Let radius r =6
The formula for area:
Area = PI. r2
=3.14159 x 62
=3.14159 x 36
=113.09724
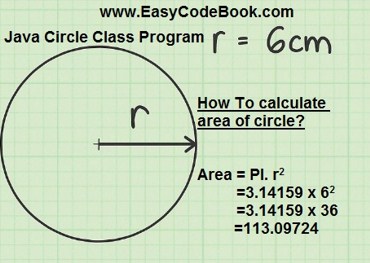
How to calculate area in Circle Java Program
The Source code of Define Circle Class in Java with Radius and Area
/* * Write a Java Program to define a Circle class with the following specification details: 1. A field radius of type double 2. a constant PI with value 3.14159 4. A no arg constructor to set radius to 0.0 5. A parameterized constructor with one parameter to assign a passed value to radius. 6. setRadius() : A setter method (also known as mutator method) for radius field 7. getRadius(): A setter method ( also known as accessor method for radis) 8. getArea(): calculates and returns area of circle Write a CircleTest class with the main() method to use the Circle class. */ package circletest; /** * * @author www.EasyCodeBook.com (c) */ class Circle { // How to define a constant in Java private final double PI = 3.14159; // radius of circle private double radius; // no arg Constructor public Circle() { radius = 0.0; } // Parameterized constructor public Circle(double r) { radius = r; } //Setter (Mutator) method for radius public void setRadius(double r) { radius = r; } //Getter (Accessor) method for radius public double getRadius() { return radius; } // method to calculate and return area public double getArea() { return PI * radius * radius; } } public class CircleTest { public static void main(String[] args) { //create first circle object with //no arg constructor Circle circle1 = new Circle(); // Create a second circle object with //parameterized constructor Circle circle2 = new Circle(3.5); //call getArea() method for object circle1 System.out.println("Area of circle for first object circle1 with radius 0="+circle1.getArea()); //call getArea() method for object circle2 System.out.println("Area of circle for second object circle2="+circle2.getArea()); //set radius of first circle to 1.5 circle1.setRadius(1.5); //once again call getArea() method for object circle1 System.out.println("Area of circle for first object circle1 with radius 1.5="+circle1.getArea()); } }
Output of the Java Circle Class Test Program
Area of circle for first object circle1 with radius 0=0.0 Area of circle for second object circle2=38.4844775 Area of circle for first object circle1 with radius 1.5=7.068577499999999
You may also like to read a Java Program to define Rectangle class.