Q: Write down a C Program to Search Multiple Records of Given Name in Binary File.
As we have seen earlier a simple version of this program to search the first record with given name in a binary file. This program will show all such records having the given name of student in a binary file.
Since, there can be many students of the same name in a class or college.
The Main Logic Behind C Program to Search Multiple Records
As you can see that we have used a while loop to read records from a binary file. We used a break statement to terminate the while loop when a record is found with given name.
Now in finding multiple records we must search in all records. Therefore, we will remove break statement. Similarly, we have used a flag variable called “found”. We set this variable to 1 if a record is found. We can modify our C code to “found++;” instead of “found=1”. So that it can show the exact number of records found according to the given criteria.
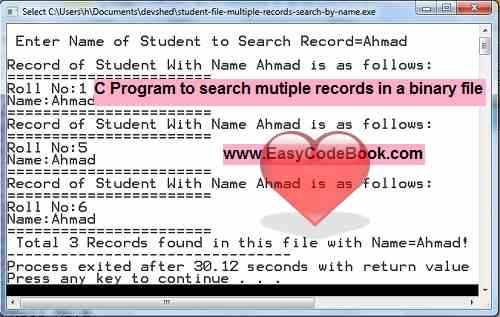
The Source Code for Searching Multiple Records with given criteria
/* Write a C program to input a name then search the multiple records of this student name in a binary file */ #include<stdio.h> #include<conio.h> #include<stdlib.h> #include<string.h> struct student { int rollno; char name[30]; }srecord; int main() { FILE *fptr; int found=0; char sname[30]; /*open binary file in read mode*/ fptr=fopen("d://sfile.dat","rb"); if(fptr==NULL) { printf("File could not open"); exit(0); } printf("\n Enter Name of Student to Search Record="); gets(sname); while((fread(&srecord,sizeof(srecord),1,fptr)==1)) { if(strcmp(srecord.name,sname)==0) { found++; printf("\nRecord of Student With Name %s is as follows:",sname); printf("\n======================="); printf("\nRoll No:%d",srecord.rollno); printf("\nName:%s",srecord.name); printf("\n======================="); } } if(found==0) printf("\n Record Not found in this file!"); else printf("\n Total %d Records found in this file with Name=%s!",found,sname); fclose(fptr); return 0; }
Pingback: C Program to Search a Record by Name in Binary File » EasyCodeBook.com
Pingback: Display Yearly Calendar C Program | EasyCodeBook.com