In this Python tutorial we will discuss Python if statement with Syntax and Examples.
Definition of Python if statement: Syntax & Example
if statement is used to decide whether a block of one or more statements will be executed or not, on the basis of a given test condition.
Syntax of Python if Statement
Syntax of simple if statement is as follows:
if condition: statement-1 statement-2 ... statement-nWhere the block of statements from statement-1 to statement-n is called if-block. It is executed, if the given condition is true, otherwise it is ignored. In second case, the control will move on the next statement after if block. Note: It is very important to note that Python uses the colon symbol ( : ) and indentation for showing where blocks of code begin and end. Therefore, if-block is just after a colon symbol and is indented by 4 spaces. Indentation of 4 spaces is a standard in Python. Hence if-block is statement-1 statement-2 … statement-n
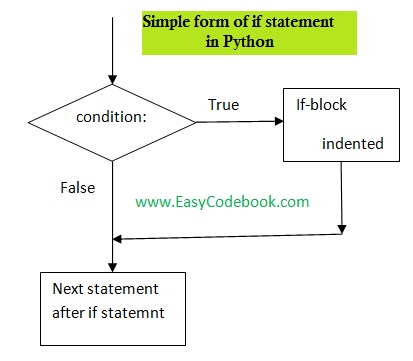
Example 1 of simple if statement in Python
Here is an if statement to show ‘Number is positive’, if number is greater than zeronum=10 if num>0: print('Number is positive')
In the above statement, if-block consists of only one statement. This print statement will be executed since 10 is greater than zero. The output will be: Number is positive
Second Example of if statement
Here we consider an if statement that will execute three print statements to show This is start of if-block Number is positive This is last statement of if-block if the given condition is true. Moreover, it will also show a message to show execution of the next statement to the if statement. ‘This is the next statement after if statement’
num=10 if num>0: print('This is start of if-block') print('Number is positive') print('This is last statement of if-block') print('This statement is outside if-block. Actually it is next statement')The output will:
This is start of if-block Number is positive This is last statement of if-block This statement is outside if-block. Actually it is next statement
Third Example of if statement (condition is false)
num=-2 if num>0: print('This is start of if-block') print('Number is positive') print('This is last statement of if-block') print('This statement is outside if-block. Actually it is next statement')Now since num is -2 which is not greater than zero, therefore the given condition is false. So, if-block will not be executed. Hence, the control will move to the next statement after if statement. The output will be as follows:
This statement is outside if-block. Actually it is next statement
Limitations in simple if statement
Actually this syntax show only a single way selection. The if-block of statements is executed or not. We have to use two conditions if we wish to check a number is even or odd.num=10 if num%2==0: print('Number is even') if num%2==1: print('Number is odd')The output will be: Number is even The reason is that % operator is called Remainder operator. 10 will be divided by two and remainder will be returned which is zero. Therefore the given condition is true. Hence, ‘Number is even’ will be printed on the screen. The second if statement will ignore its if-block, since the condition num%2==1 is false. Consider again:
num=11 if num%2==0: print('Number is even') if num%2==1: print('Number is odd') The output will be: Number is odd Since the condition of second if statement is true so if-bock of second if statement is executed.
Working of if Statement
First of all the given condition is evaluated. If the given test gives a true result, then the block of statements is executed. If the result of the test condition is false, then the block of statements is not executed – that is ignored.