Topic: Python file Phonebook
Python file Phonebook Program: Write a Python Program using text files to create and maintain a Phone Book.
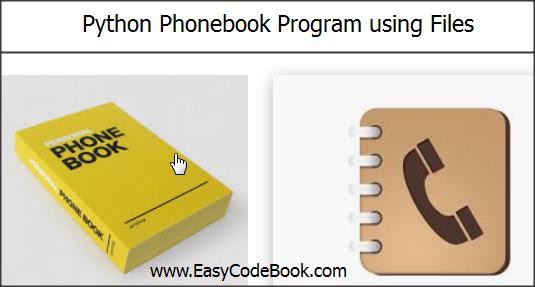
Python File Phonebook Program
Python Phone book Program uses file to provide the following functions:
- You can add new contact records
- It will display all the contact records stored already
- Search function will provide you the searching option to search any of the stored contacts from the phone book.
The Phone Book will display the following Menu and will support
the corresponding functionality:
*** Phone Book Menu ***
Enter 1,2,3 or 4:
Enter 1 To Display Your Contacts Records
Enter 2 To Add a New Contact Record a new contact
Enter 3 To search your contacts
Enter 4 To Quit
**********************
Note(update: May 29, 2021): A Short and better version of Python phone book program is added at the end of this post.
''' Write a Python Program using text files to create and maintain a Phone Book. The Phone Book will display the following Menu and will support the corresponding functionality *** Phone Book Menu *** Enter 1,2,3 or 4: Enter 1 To Display Your Contacts Records Enter 2 To Add a New Contact Record a new contact Enter 3 To search your contacts Enter 4 To Quit ********************** author: www.Easycodebook.com (c) ''' file_name = "d://phonebook.txt" file1 = open(file_name, "a+") file1.close def show_main_menu(): ''' Show main menu for Phone Book Program ''' print("\n *** Phone Book Menu ***\n"+ "author: www.Easycodebook.com (c)\n"+ "------------------------------------------\n"+ "Enter 1,2,3 or 4:\n"+ "Enter 1 To Display Your Contacts Records\n" + "Enter 2 To Add a New Contact Record\n"+ "Enter 3 To search your contacts\n"+ "Enter 4 To Quit\n**********************") choice = input("Enter your choice: ") if choice == "1": file1 = open(file_name, "r+") file_contents = file1.read() if len(file_contents) == 0: print("Phone Book is empty") else: print (file_contents) file1.close ent = input("Press Enter to continue ...") show_main_menu() elif choice == "2": enter_contact_record() ent = input("Press Enter to continue ...") show_main_menu() elif choice == "3": search_contact_record() ent = input("Press Enter to continue ...") show_main_menu() elif choice== "4": print("Thanks for using Phone Book Program presented by:"+ "\nwww.EasyCodeBook.com "+"\nFor Perfect Programming Tutorials: Python, Java, C, C++") else: print("Wrong choice, Please Enter [1 to 4]\n") ent = input("Press Enter to continue ...") show_main_menu() def search_contact_record(): ''' This function is used to searches a specific contact record ''' search_name = input("Enter First name for Searching contact record: ") rem_name = search_name[1:] first_char = search_name[0] search_name = first_char.upper() + rem_name file1 = open(file_name, "r+") file_contents = file1.readlines() found = False for line in file_contents: if search_name in line: print("Your Required Contact Record is:", end=" ") print (line) found=True break if found == False: print("There's no contact Record in Phone Book with name = " + search_name ) def input_fname(): ''' Converts first letter of your name to Upper case ''' fname = input("Enter First name ") rem_fname = fname[1:] first_char = fname[0] return first_char.upper() + rem_fname def input_lname(): ''' Converts first letter of last name to Upper case ''' lname = input("Enter Last name ") rem_lname = lname[1:] first_char = lname[0] return first_char.upper() + rem_lname def enter_contact_record(): ''' It collects contact info firstname, last name, email and phone ''' first = input_fname() last = input_lname() phone = input('Enter Phone number ') email = input('Enter E-mail ') contact = ("[" + first + " " + last + ", " + phone + ", " + email + "]\n") file1 = open(file_name, "a") file1.write(contact) print( "This contact\n " + contact + "has been added successfully!") show_main_menu()
Output of A Sample Run of Python Phonebook Program using File
Note: The output will be in a single color:D
*** Phone Book Menu *** author: www.Easycodebook.com (c) ------------------------------------------ Enter 1,2,3 or 4: Enter 1 To Display Your Contacts Records Enter 2 To Add a New Contact Record Enter 3 To search your contacts Enter 4 To Quit ********************** Enter your choice: 1 [Ahmad Naveed, 0300-776767, ahmad@gmail.com] [Toqir Nasir, 0334-789777, asad@gmail.com] [Nasir Ahmad, 03001234567, nasir@gmail.com] [Javed Iqbal, 0334-75009876, javed1bal@yahoo.com] Press Enter to continue ... *** Phone Book Menu *** author: www.Easycodebook.com (c) ------------------------------------------ Enter 1,2,3 or 4: Enter 1 To Display Your Contacts Records Enter 2 To Add a New Contact Record Enter 3 To search your contacts Enter 4 To Quit ********************** Enter your choice: 2 Enter First name zahoor Enter Last name khan Enter Phone number 03006743249 Enter E-mail zakhan@gmail.com This contact [Zahoor Khan, 03006743249, zakhan@gmail.com] has been added successfully! Press Enter to continue ... *** Phone Book Menu *** author: www.Easycodebook.com (c) ------------------------------------------ Enter 1,2,3 or 4: Enter 1 To Display Your Contacts Records Enter 2 To Add a New Contact Record Enter 3 To search your contacts Enter 4 To Quit ********************** Enter your choice: 3 Enter First name for Searching contact record: zahoor Your Required Contact Record is: [Zahoor Khan, 03006743249, zakhan@gmail.com] Press Enter to continue ... *** Phone Book Menu *** author: www.Easycodebook.com (c) ------------------------------------------ Enter 1,2,3 or 4: Enter 1 To Display Your Contacts Records Enter 2 To Add a New Contact Record Enter 3 To search your contacts Enter 4 To Quit ********************** Enter your choice: 555 Wrong choice, Please Enter [1 to 4] Press Enter to continue ... *** Phone Book Menu *** author: www.Easycodebook.com (c) ------------------------------------------ Enter 1,2,3 or 4: Enter 1 To Display Your Contacts Records Enter 2 To Add a New Contact Record Enter 3 To search your contacts Enter 4 To Quit ********************** Enter your choice: 4 Thanks for using Phone Book Program presented by: www.EasyCodeBook.com For Perfect Programming Tutorials: Python, Java, C, C++
Update: 29-05-2021
A Shorter and Better Version
''' Write a Python Program using text files to create and maintain a Phone Book. The Phone Book will display the following Menu and will support the corresponding functionality *** Phone Book Menu *** Enter 1,2,3 or 4: Enter 1 To Display Your Contacts Records Enter 2 To Add a New Contact Record a new contact Enter 3 To search your contacts Enter 4 To Quit ********************** author: www.Easycodebook.com (c) ''' file_name = "d://phonebook.txt" file1 = open(file_name, "a+") file1.close def show_main_menu(): ''' Show main menu for Phone Book Program ''' print("\n *** Phone Book Menu ***\n"+ "author: www.Easycodebook.com (c)\n"+ "------------------------------------------\n"+ "Enter 1,2,3 or 4:\n"+ "Enter 1 To Display Your Contacts Records\n" + "Enter 2 To Add a New Contact Record\n"+ "Enter 3 To Search your contacts\n"+ "Enter 4 To Quit\n**********************") choice = input("Enter your choice: ") if choice == "1": file1 = open(file_name, "r+") file_contents = file1.read() if len(file_contents) == 0: print("Phone Book is empty") else: print (file_contents) file1.close ent = input("Press Enter to continue ...") show_main_menu() elif choice == "2": enter_contact_record() ent = input("Press Enter to continue ...") show_main_menu() elif choice == "3": search_contact_record() ent = input("Press Enter to continue ...") show_main_menu() elif choice== "4": print("Thanks for using Phone Book Program presented by:"+ "\nwww.EasyCodeBook.com "+"\nFor Perfect Programming Tutorials: Python, Java, C, C++") else: print("Wrong choice, Please Enter [1 to 4]\n") ent = input("Press Enter to continue ...") show_main_menu() def search_contact_record(): ''' This function is used to searches a specific contact record ''' search_name = input("Enter First name for Searching contact record: ") search_name = search_name.title() file1 = open(file_name, "r+") file_contents = file1.readlines() found = False for line in file_contents: if search_name in line: print("Your Required Contact Record is:", end=" ") print (line) found=True break if found == False: print("There's no contact Record in Phone Book with name = " + search_name ) def enter_contact_record(): ''' It collects contact info firstname, last name, email and phone ''' first = input('Enter First Name: ') first = first.title() last = input('Enter Last Name: ') last = last.title() phone = input('Enter Phone number: ') email = input('Enter E-mail: ') contact = ("[" + first + " " + last + ", " + phone + ", " + email + "]\n") file1 = open(file_name, "a") file1.write(contact) print( "This contact\n " + contact + "has been added successfully!") show_main_menu()
You will also like:
- Python File Copy Program using shutil.filecopy
- Find Occurrences of Each Word in Text File Python Program
- Count Words in Each Line of Text File Python Program
- Count Words in Text File Python Program
- Count Lines in Text File Python Program
and more programs on Files
- Copy All Records to Another File Program in C Plus Plus
- Menu Driven C++ Program To Read and Write in Binary File
and some more File programs in C Language
- C File Program to Save Records in a Binary File
- C Program to Read Records From Binary File
- C Program to Append / Add More Records in Binary File
- C Program to Search a Record in Binary File C Programming
- C Program to Search a Record by Name in Binary File
- C Program to Search Multiple Records of Given Name in Binary File
- C++ Program to Calculate Bonus on Salary Grade Wise
nice job, I love easycodebook.com !
https://easycodebook.com/2020/04/python-file-phonebook-program/