Python Class and Objects with Program Examples
Python Class and Objects with Program Examples
- Python Object Oriented Programming
- Class and Objects
- What is a class
- What is an object
- How to define a class
- How to create an object
- Instance Variables
- How To Define Instance variables
- Example of Instance variables
- What is a Constructor in Python Classes?
- What are Methods
- How to Define a Method
- Example of Method Definition
- Class Variables
- Example of Class Variable
- How to access a class variable
- Creating a Student class in Python
- Creating a Math1 class to add two numbers in Python
- Python Circle Class With Getter Setter Methods
- Python Create New Class Rectangle
- Python Class and Objects With Code Examples
Python Class and Objects with Program Examples
Topic: Python Class and Objects with Program Examples
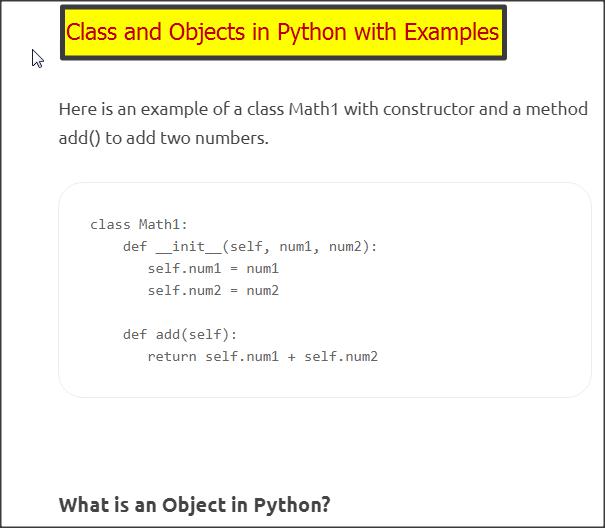
Definition of Class in Python
A class is a template to create objects or we can say that a class is a blueprint to create instances. A class is a design plan to create objects. For example Student is a class and all the students with different names say Ahmad, Niaz, Tariq and Zahoor etc are the objects or instances of the Student class. Similarly Sheep is a class and Dolly is an object or instance.
Importance of Class in Object Oriented Programming
Classes are used to define new data types with the required set of values and operations defined on those values. Every class generally defines a set of Instance Variables and Instance Methods. These instance variables and methods define the state and actions of an object of that particular class.
Actually, a class will provide a mechanism to bundle the data and functionality together. Creating a new class creates a new type of object, allowing new instances of that type to be made. Each class instance can have attributes attached to it for maintaining its state.
How To Define a Class in Python?
The "class" keyword is used to start a class definition.
General syntax is:
class ClassName: <statement-1> . . . <statement-N> Here statements are normally method definitions.
Example of a classs
Here is an example of a class Math1 with a parameterized constructor containing instance variable initialization and a method add() to add two numbers.
class Math1: def __init__(self, num1, num2): self.num1 = num1 self.num2 = num2 def add(self): return self.num1 + self.num2
Explanation of Class Definition Syntax
class Keyword
First of all we write a keyword class which is followed by a class name and a colon.
Constructor
There is a parameterized constructor which is a special method to start with __init__ . It contains three parameters , the first parameter is generally self which points to the current object. Other two parameters num1 and num2 are instance variables.
Method
Next comes an instance method to add two numbers and return the result.
What is an Object in Python?
An object is an instance of a class.
Foe example the construction plan for a house is an example of a class. Whereas a built house with the help of construction design plan is an example of its object.
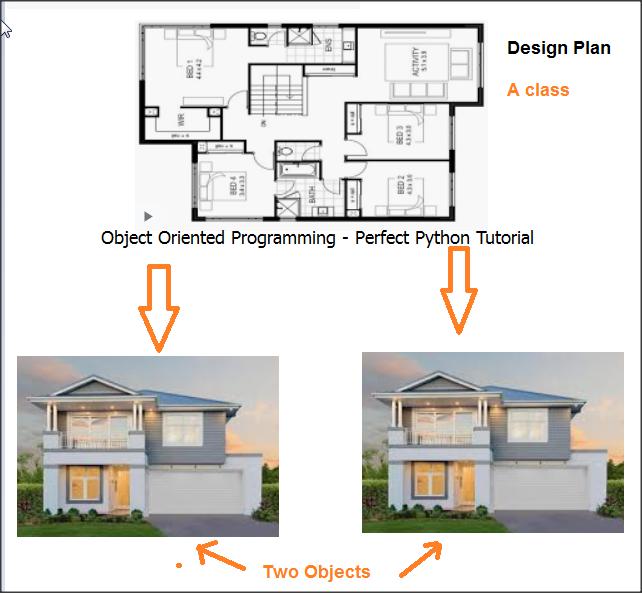
Python Class and Objects with example programs
In Python, a class will provide a means of bundling data and functionality together. we create a class to create a new type of object. Now we can use this class to create new objects or instances of the defined class.
How to create an object of a class
General syntax is:
object_ref_variable = ClassName()
or
object_ref_variable = ClassName(argument_list)
Example:
stu1=Student("Ahmad",18) # create first student object
stu2 = Student("Jameel",20) # create second student object
What are attributes of an Object?
Each class instance or object may have attributes. These attributes are used to maintain the state of the object. These attributes contains data of the object in different variables.
What is an Instance Variable?
An instance variable is the variable belonging to an object.Instance variables are declared within methods (functions defined within a class are called methods). Each object can store its own set of data into its instance variables.
For example, consider a class Math1 which uses two number variables num1 and num2. If we create two objects from Math1 class, named obj1 and obj2. Now obj1 will have its own num1, num2, say with values 10 and 20. Similarly the second object obj2 will have its own set of instance variables num1, num2, say with values 500 and 1000.
Example of Instance Variables
For example, consider a class Math1 to perform addition of two numbers num1 and num2. Here num1 and num2 are called instance variables.
How To Define Instance Variables?
We can define instance variables within the special method __init__() or constructor method. For example, we will define instance variables num1 and num2 in a constructor which is a special method named __init()__ as given below:
class Math1: def __init__(self, num1, num2) self.num1 = num1 self.num2 = num2
How Instance variables are accessed?
The instance variables are accessed using object name or "self" with dot notation
self.num1 = num1
self.num2 = num2
print("\nName:",self.name,"\nAge:",self.age,")
What is a Constructor in Python Classes?
A constructor is a special method of a class which is executed automatically when an object is created. A constructor is used to intialize the instance variables of the object of a class. The name of a constructor is always __init__. The first parameter of a constructor or any other method is always 'self'. The 'sel'f is a special variable to refer to the current class instance or object.
Example of Constructor in a Class
for example we will define a constructor for a class called Math1.
class Math1: def __init__(self, num1, num2) self.num1 = num1 self.num2 = num2
Here we will use three arguments named self, num1, num2. The first argument to every method in your class is a special variable called self . Note that whenever your class refers to one of its variables or methods, it must precede them by self, for example:
self.num1 = num1
self.num2 = num2
The other two arguments num1 and num2 will give values to two instance variables of an object. That is they will initialize the state of the object.
What are Methods of an Object?
The Methods are the functions defined within a class. These methods perform some actions on the data of the objects of that class.
Creating a new class creates a new type of object, allowing new instances of that type to be made. Each class instance can have attributes attached to it for maintaining its state. Class instances can also have methods (defined by its class) for modifying its state.
Example of a Method definition in Python
Here is an example of a class Math1 with constructor and a method add() to add two numbers.
class Math1: def __init__(self, num1, num2): self.num1 = num1 self.num2 = num2 def add(self): return self.num1 + self.num2
How we call a Method?
We can call a method with object name and dot notation:
stu1.show_data()
What are Class Variables?
A variable which is defined within a class but outside of any methods, is called a class variable. It is a class level variable. It is used to store a common data for all objects of that class. A class variable has the same value for all objects. For example, If we define a class Student as follows:
class Student: college="KFGC - RYK" def __init__(self,name,age): self.name=name self.age=age def show_data(self): print("\nName:",self.name,"\nAge:",self.age,"\nCollege:",Student.college)
Note that here, there are two instance variables name and age.
Whereas, college variable is a class variable. A class variable is normally initialized at the time of definition. Now since this Python program is for all students of a particular college, so we have defined a class variable named college with value "KFGC - RYK". This class variable is common to all objects of Student class.
How we can access a class variable
This class variable can be accessed with object as well as with class name.
For example, print(Student.college) will print KFGC - RYK.
The complete Program for Student class in Python
class Student: college="KFGC - RYK" def __init__(self,name,age): self.name=name self.age=age def show_data(self): print("\nName:",self.name,"\nAge:",self.age,"\nCollege:",Student.college) # create two objects for two students stu1=Student("Ahmad",18) stu2 = Student("Jameel",20) # call show_data() method for both objects stu1.show_data() stu2.show_data()
Output:
Name: Ahmad Age: 18 College: KFGC - RYK Name: Jameel Age: 20 College: KFGC - RYK
Python Class and Objects with Program Examples
Python Create New Class Rectangle
Write a Python Program to design a new class Math1