Task: Menu Driven ( C++ ) C Plus Plus Program To Read and Write in Binary File. How This C++ File Program Works? This C++ program uses a menu driven approach.
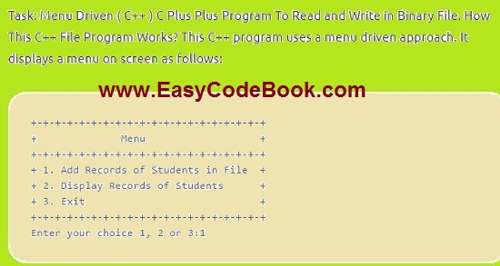
Menu Driven ( C++ ) C Plus Plus Program To Read and Write in Binary File.
It displays a menu on screen as follows:
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ + Menu + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ + 1. Add Records of Students in File + + 2. Display Records of Students + + 3. Exit + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ Enter your choice 1, 2 or 3:1
As you can see, the C++ filing Program is asking the user to enter his choice 1, 2 or 3. If the user enters 1 and press Enter key, this will start ‘adding records process’.
*******Adding Records********* Enter Student Record Roll No:1 Name:Ahmad Record has been added successfully Add more records? press y or n =y Enter Student Record Roll No:2 Name:Shahid Record has been added successfully Add more records? press y or n =n
Therefore, the user adds two records and then he enters n to stop adding records process. This will display the menu again on screen. Further, if the user enters 2 as his choice, the program will show all ( that is 2) records on screen. This program will display the menu again. If the user enters 3 then the program will be terminated.
The Source Code of this Menu Driven C++ Program To Read and Write in Binary File
/* ====================================================*/ /* */ /* */ /* (c) 2019 Author: wwww.EasyCodeBook.com */ /* */ /* Description */ /* Write a C++ Program to read and write records in */ /* binary file. Use a menu driven approach */ /* */ /* ====================================================*/ #include<iostream> #include<fstream> #include<conio.h> #include<stdlib.h> #include<string> using namespace std; void addRecords(); void displayRecords(); struct student { int rollno; char name[30]; }srecord; fstream file1; int main() { int choice=1; while(1) { // prnit menu cout<<"\n"; cout<<"+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+\n"; cout<<"+ Menu +\n"; cout<<"+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+\n"; cout<<"+ 1. Add Records of Students in File +\n"; cout<<"+ 2. Display Records of Students +\n"; cout<<"+ 3. Exit +\n"; cout<<"+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+\n"; cout<<"Enter your choice 1, 2 or 3:"; cin>>choice; if(choice==1) addRecords(); else if (choice==2) displayRecords(); else if (choice==3) break; else cout<<"\n Invalid choice, Enter 1, 2 or 3 only:"; } cout<<"\nThanks for visiting www.EasyCodeBook.com"; } void addRecords() { char ans; int i; //opening binary file in appending / writing mode file1.open("d://cppfile.dat",ios::binary |ios::app); if(!file1) { cout<<"File could not open"; exit(0); } cout<<"\n*******Adding Records*********\n"; while(1) { cout<<"\nEnter Student Record\n"; cout<<"Roll No:"; cin>>srecord.rollno; getchar(); cout<<"Name:"; gets(srecord.name); /* write a record to binary file */ file1.write((char*)&srecord,sizeof(srecord)); cout<<"\nRecord has been added successfully"; cout<<"\nAdd more records? press y or n ="; ans=getche(); if(ans=='n' || ans=='N') { file1.close(); break; } } } void displayRecords() { int i; //opening binary file in reading mode file1.open("d://cppfile.dat",ios::binary |ios::in); if(!file1) { cout<<"File could not open"; exit(0); } cout<<"\n***Reading & Displaying Records***\n"; while(!file1.eof()) { file1.read((char*)&srecord,sizeof(srecord)); if(!file1.eof()) { cout<<"\n------------------\n"; cout<<"Roll No:"<<srecord.rollno; cout<<"\nName:"<<srecord.name; } } file1.close(); }
A Sample Run with Output of Menu Driven C++ Program To Read and Write in Binary File
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ + Menu + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ + 1. Add Records of Students in File + + 2. Display Records of Students + + 3. Exit + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ Enter your choice 1, 2 or 3:1 *******Adding Records********* Enter Student Record Roll No:1 Name:Ahmad Record has been added successfully Add more records? press y or n =y Enter Student Record Roll No:2 Name:Shahid Record has been added successfully Add more records? press y or n =n +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ + Menu + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ + 1. Add Records of Students in File + + 2. Display Records of Students + + 3. Exit + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ Enter your choice 1, 2 or 3:2 ***Reading & Displaying Records*** ------------------ Roll No:1 Name:Ahmad ------------------ Roll No:2 Name:Shahid +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ + Menu + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ + 1. Add Records of Students in File + + 2. Display Records of Students + + 3. Exit + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ Enter your choice 1, 2 or 3:3 Thanks for visiting www.EasyCodeBook.com -------------------------------- Process exited after 1017 seconds with return value 0 Press any key to continue . . .