Task: Write a Java Program To Mark Even and Odd Numbers in Array. First of all the user will enter the size of the array for example 10 or 20 etc. The program will ask the user to enter n numbers according to the given size of the array.
The user will enter n numbers one by one. The Java program will display each number in the array with an Even or Odd message.
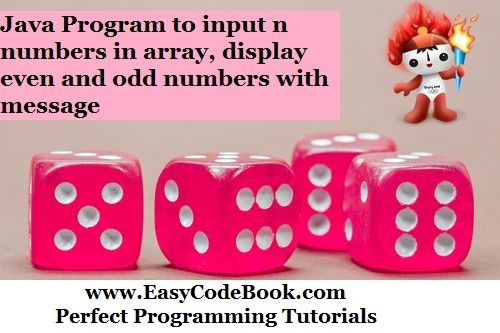
The Java Source Code
/* * Write a Java Program to input n numbers in array * and Mark Even and Odd Numbers. */ package evenoddarray; /** * * @author www.EasyCodeBook.com */ import java.util.Scanner; public class EvenOddArray { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter the size of array="); int siz = input.nextInt(); int arr[] = new int[siz]; for (int i = 0; i < siz; i++) { System.out.print("Enter the number in array="); arr[i] = input.nextInt(); } System.out.println("Even and Odd numbers in array are:"); for (int i = 0; i < siz; i++) { if (arr[i] % 2 == 0) System.out.println(arr[i] + " is even"); else System.out.println(arr[i] + "is odd"); } } }
The output of a sample run of the Java Array program
Enter the size of array=10 Enter the number in array=11 Enter the number in array=23 Enter the number in array=22 Enter the number in array=45 Enter the number in array=56 Enter the number in array=67 Enter the number in array=68 Enter the number in array=112 Enter the number in array=678 Enter the number in array=90 Even and Odd numbers in array are: 11is odd 23is odd 22 is even 45is odd 56 is even 67is odd 68 is even 112 is even 678 is even 90 is even Another Sample Run Enter the size of array=5 Enter the number in array=4567 Enter the number in array=7890 Enter the number in array=1234 Enter the number in array=2345 Enter the number in array=54321 Even and Odd numbers in array are: 4567is odd 7890 is even 1234 is even 2345is odd 54321is odd