Tpoic: List in Python With Examples
What is List in Python Language?
List is a dynamic collection of items of different types. Lists contain utems similar to the arrays of other programming languages like C, C++ and Java etc.
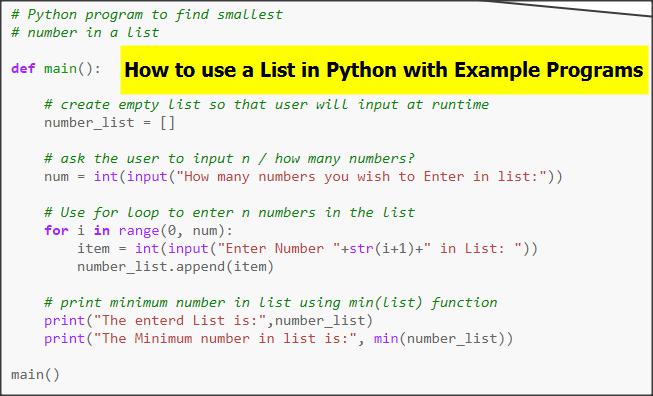
List in Python Language With Example Programs
Difference between Array and List
The array is the collection of items of same data type. That is arrays are homogenious in nature. But Lists can contain items of different types, for example we can define a list of integers, floats and strings at the same time. Therefore Lists are heterogenious in nature.
Similarly, most of the programming languages support static arrays. The size of a static array cannt be changed during execution. Whereas we can change the siz of a list in Python language as and when needed during the exection of the program.
How To Create a List in Python Language
List is created by placing a sequence of items separeted by commas in a pair of square brackets.
Example of a List of 5 Integers
int_list = [10,20,11,2,90]
How to Create a List of Floats
temperatures = [32.7, 40.0, 39.5, 38.6, 41.4]
How to Create a List of Strings
my_strings = [‘hello’, ‘ Python’, ‘ Programming’, ‘ World’]
How to create an Empty List
my_list = [ ]
How to Add Items / Elements in List
We will use append() method to add a single item or element in the list.
Example:
# create a list named list1 as empty list list1 = [ ] # add item 900,1100,15 one by one list1.append(900) list1.append(1100) list1.append(15) # print the list print(list1) # print size / length of list print ('Size of list=',len(list1))
Output: [900, 1100, 15] Size of list= 3
Adding an element at a specified location
insert() method is used to add an elemnt / item on a given location of the list.
insert() method uses two arguments, index and the item to be inserted.
For example to add 500 on index = 1 , that is on location 2 of list, we will use:
list1.insert(1,500)
In this way, if a list is [900, 1100, 15] with
Size of list= 3, after execution of the above insert(1,500) method call, the list will take the form:
[900, 500, 1100, 15] with Size of list= 4
# create a list named list1 as empty list list1 = [ ] # add one item 900 list1.append(900) list1.append(1100) list1.append(15) # print the list print(list1) # print size / length of list print ('Size of list=',len(list1)) # Insert element 500 on index 1 list1.insert(1,500) # print the list print(list1) # print size / length of list print ('Size of list=',len(list1))
Output: [900, 1100, 15] Size of list= 3 [900, 500, 1100, 15] Size of list= 4
How to Acess a List in Python Langauge
As we know that a List in Python starts with index zero to size-1 as the index of the last item in the list. We can use index to access a list. For example to access first item we will use:
print(list1[0]) and to print the last item we will use print(list1[3]).
How to print a List?
We can print the entire list by using the name of the list:
list1 = [10,20,500]
print(list1)
Output:
[10,20,500]
We can use a for each loop for sequences to print the all items of List:
list1 = [10,20,500] for item in list1: print(item) Output: 10 20 500
We can use for loop with range() function to display the whole list:
list1 = [10,20,500] for i in range(0,len(list1)): print(list1[i]) Output: 10 20 500
Using Negative Index in Python Lists
We can also use negative index to access elements of a Python List. We can access the list in reverse order. For example to display the last item of the list we can use:
print(list1[-1])
this will print 500, the last item. Similarly, print(list1[-2]) will print 20 which the second last item.
More Examples of Negative Indexing in Python List
#Python Code
prog_languages = [‘Python’, ‘Jave’, ‘C++’]
print(‘Last element of List is=’,prog_languages[-1]);
print(“Negative Indexing Demo”)
Output:
Last element of List is= C++
Negative Indexing Demo
Printing a List in Reverse Order by Slicing
print(list1[::-1])
# Python program to show List slicing # create a list named list1 list1 = [900,1100,15] # print the list print('List = ', list1) # print size / length of list print ('Size of list=',len(list1)) # print last item . use negative indexing print('Last item=',list1[-1]) # print second last item of list print('Second Last item=',list1[-2]) # print all items in reverse order print('List in Reverse order=',list1[::-1])
Output: List = [900, 1100, 15] Size of list= 3 Last item= 15 Second Last item= 1100 List in Reverse order= [15, 1100, 900]
Changing the Items in a List
Changing List Items in Python
We can change list items by using index and assignment operator.
For example to change the first item in list we will use:
my_list[0]=100
Example Python Code:
my_list=[100,300,300,400]
#now we change second item as 200
my_list[1]=200
print( my_list)
Output:
[100, 200, 300, 400]
How to input items in List at runtime from User
We will create an empty list and use the input() function to get items from the user. The append() method is used to enter items at runtime.
def main(): # create empty list so that user will input at runtime number_list = [] # ask the user to input n / how many numbers? num = int(input("How many numbers you wish to Enter in list:")) # Use for loop to enter n numbers in the list for i in range(0, num): item = int(input("Enter Number "+str(i+1)+" in List: ")) number_list.append(item) # print the list print("The enterd List is:",number_list) main()
Output: How many numbers you wish to Enter in list:5 Enter Number 1 in List: 134 Enter Number 2 in List: 23 Enter Number 3 in List: 45 Enter Number 4 in List: 1 Enter Number 5 in List: 7890 The enterd List is: [134, 23, 45, 1, 7890]
How to use pop() method to remove and return an item by index
list1 = [10, 20, 30, 40, 50] # Remove first item item = list1.pop(0) print('Item removed or popped=',item) print('Remaining list=',list1)
Item removed or popped= 10 Remaining list= [20, 30, 40, 50]
Using remove(item_value) Method to remove an item by value
list1 = [10, 20, 30, 40, 50] print('List is: ', list1) # Remove item with value 40 list1.remove(40) print('Remaining list=',list1)
Output: List is: [10, 20, 30, 40, 50] Remaining list= [10, 20, 30, 50]
How to Remove All Items of List in Python Language?
We will use clear() method to delete all items of list.
list1 = [10, 20, 30, 40, 50] print('List is: ', list1) # Remove all items list1.clear() print('Remaining list=',list1)
Output:
List is: [10, 20, 30, 40, 50]
Remaining list= []
How To Copy a List in Python
We copy a Python list using the copy() method. The copy() method will copy the contents a a list into a new list.
Syntax:
list2 = list1.copy()
Example:
Note: If we assign a list to another list, it just copies the reference to the list, not the actual contents of list. That is both lists will refer to the same list. Therefore we will use copy() method to copy a list into another list.
Example Programs using List in Python Language
Pingback: Python Counting Words Occurrences | EasyCodeBook.com