Task – Java Check Armstrong Number Program. This Java Programming Tutorial will explain the source code of Armstrong Number checking program. The Java program will ask you to enter an integer number (whole number). When you will enter a number, this Java Check Armstrong Number program will find whether the given number is an Armstrong number or not.
What is an Armstrong Number?
An armstrong Number of N digits is an integre such that the sum of its every digit raised to the power N is equal to the number itself.
For example, consider the integer number 153. Since, in this case N=3 which is the number of digits in 153.
Hence the sum of digits 1,5,3 raised to the power 3 is calculated as:
Num = 153
Sum = 13 + 53 + 33
Hence
Sum = 1 + 125 + 27
Sum = 153
Since, we find that Num is equal to the Sum of cubes of the digits, therefore, we conclude that 153 is an Armstrong Number. Because the sum of cubes of its digits is equal to the number itself.
An Armstrong Number of Three Digits
An Armstrong number of three digits is an integer such that the sum of the cubes of its digits is equal to the number itself. For
example, 370 is an Armstrong number since 3**3 + 7**3 + 0**3 = 370. Note that ‘**’ represent power operation.
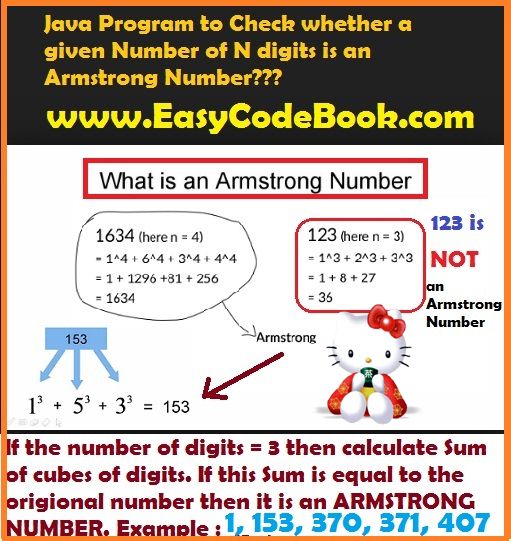
Java Armstrong Number Check Program
The Source Code of Java Check Armstrong Number Program
/* * Write a Java program to input * a number and check whether it is * an Armstrong Number? */ package checkarmstrongnumber; /** public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print(“Enter a number to check for Armstrong Number:”); String s = String.valueOf(num); n = num; while (n != 0) if(num == sum) } } |
Java Check Armstrong Number Program –
Output – Different Sample Runs
Enter a number to check for Armstrong Number:1634
1634 is an Armstrong Number
Enter a number to check for Armstrong Number:153
153 is an Armstrong Number
Enter a number to check for Armstrong Number:407
407 is an Armstrong Number
Enter a number to check for Armstrong Number:456
456 is not an Armstrong Number
Enter a number to check for Armstrong Number:1
1 is an Armstrong Number
Enter a number to check for Armstrong Number:371
371 is an Armstrong Number
Enter a number to check for Armstrong Number:370
370 is an Armstrong Number
I dugg some of you post as I thought they were very useful handy