Pointer Program Maximum Array Number: Write a C++ program to input 5 numbers in array. Find maximum number in array using pointer notation.
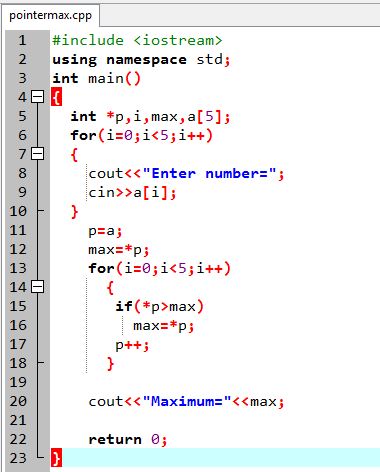
Source Code Pointer Program Maximum Array Number
Let’s go through the C++ program line by line:
#include <iostream>
This line includes the standard input-output stream library, which provides functionalities for input and output operations.
using namespace std;
This line declares that all the entities in the standard namespace std will be used in this program, so we don’t need to prefix them with std::.
int main()
{
This is the starting point of the program. main() is the entry point for all C++ programs, where execution begins.
int *p, i, max, a[5];
This line declares four variables:
p: a pointer to an integer
i: an integer used as a loop counter
max: an integer to store the maximum value
a[5]: an array of integers to store 5 values
for(i=0; i<5; i++)
{
cout << “Enter number=”;
cin >> a[i];
}
This loop iterates five times, prompting the user to enter a number each time and storing it in the a array.
p = a;
max = *p;
Here, the pointer p is assigned the address of the first element of the array a, and the variable max is initialized with the value at that address.
for(i=0; i<5; i++)
{
if(*p > max)
max = *p;
p++;
}
This loop iterates over each element of the array a using the pointer p. If the value pointed to by p is greater than max, max is updated with that value. Then, the pointer p is incremented to point to the next element.
cout << “Maximum=” << max;
Finally, the maximum value stored in max is printed to the console.
return 0;
This statement indicates that the program has executed successfully and is returning a status code of 0 to the operating system. It’s a convention to return 0 from main() to indicate success.