Python Math Operators are the special symbols to perform common mathematical operations on data called operands. The Math operators include the following:
Table Explaining the Use of Python Math Operators
Math Operator | Used for | Example | Result |
---|---|---|---|
+ | Addition | 2+9 | 11 |
– | Subtraction | 9-2 | 7 |
* | Multiplication | 7*5 | 35 |
/ | Division | 7/2 | 3.5 |
// | Integer Division | 7//2 | 3 |
% | Modulus / Remainder | 5%2 | 1 |
** | Power or Exponentiation | 2**3 | 8(2*2*2) |
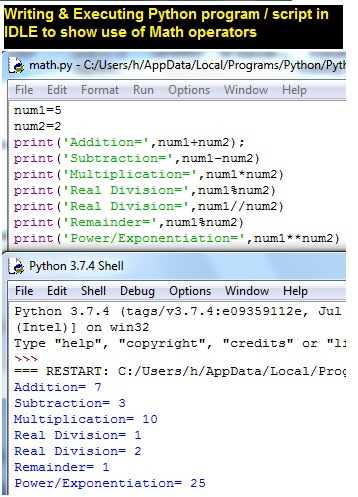
Use of Python Math operators with examples
The Integer Division Operator ( // )
The Difference between / operator and // operator in Python
The division operator / performs a real division. It gives result in floating point number. For example, dividing 7 by 2 as 7/2 will give the result 3.5.
Whereas, the integer division operator which is double slash // will give only the integer part in the result. Since, It will perform integer division and ignore the fractional part. For, example dividing 7 by 2 as 7//2 will give 3. and not 3.5. Because it will truncate the fractional part.
A Simple Program / Script for Expianing the Use of Math Operators:
num1=5 num2=2 print('Addition=',num1+num2); print('Subtraction=',num1-num2) print('Multiplication=',num1*num2) print('Real Division=',num1%num2) print('Real Division=',num1//num2) print('Remainder=',num1%num2) print('Power/Exponentiation=',num1**num2) </pre
The output: Addition= 7 Subtraction= 3 Multiplication= 10 Real Division= 1 Real Division= 2 Remainder= 1 Power/Exponentiation= 25