Task: Write a Java Array Program to Find Sum of Even Numbers in Array.
This Java program uses a simple mathematical logic to check whether an array item ( that is number ) is even. The idea is to divide a number by two and obtain remainder. If remainder is a zero then the given number is even. Moreover, whenever it finds an even number, it will add it to a variable sum with an assignment statement “sum = sum + a[i];”. Remember that a[i] is the i’th item of the array named “a”.
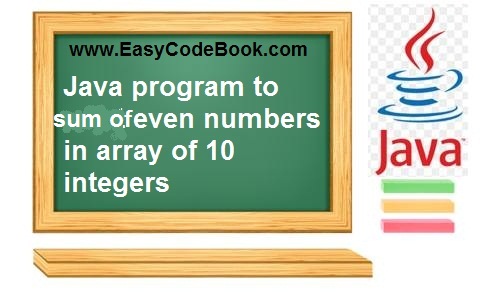
The source code for this Java Array program is as follows:
/* * Write a Java Program to input 10 integers in array and find sum of even items of array */ package arrayevensum; import java.util.Scanner; /** * @author www.EasyCodebook.com */ public class ArrayEvenSum { public static void main(String[] args) { Scanner input = new Scanner(System.in); int a[]=new int[10],i , sum=0; for(i=0;i<a.length ;i++) { System.out.print("Enter number in Array["+i+"]="); a[i]=input.nextInt(); } for(i=0;i<a.length ;i++) { if(a[i]%2==0) sum = sum+ a[i]; } System.out.println("Sum of Even Numbers in Array is:"+sum); } }
A sample run of this Java program using array without output of the program is as follows:
Enter number in Array[0]=2
Enter number in Array[1]=1
Enter number in Array[2]=2
Enter number in Array[3]=1
Enter number in Array[4]=4
Enter number in Array[5]=5
Enter number in Array[6]=6
Enter number in Array[7]=7
Enter number in Array[8]=2
Enter number in Array[9]=2
Sum of Even Numbers in Array is:18