“Four Function Calculator Program” is a C program to input first number, an operator and second number to perform required calculation. A sample input string is 2+4 [Press Enter]. The 4 function calculator program will add 2 and 4 and display the answer of addition that is 6.
The operators may be arithmetic operators for:
- addition +
- subtraction –
- multiplication *
- division /
This C program uses if else if statement to check whether operator is +, -, * or / and performs the related mathematical operation and show result to the user.
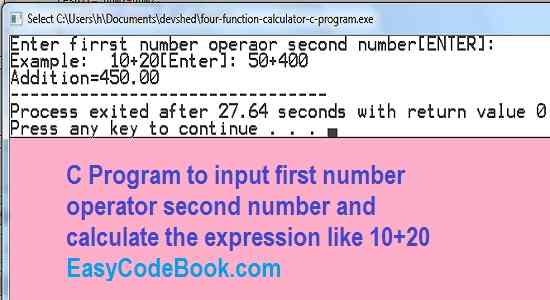
The source code for plus, minus, multiplication and division calculator in C programming language is in the following code block with syntax coloring.
/* Write a C program to work as 4 function calculator +, _, *, / Sample input string may be 20+30[Press Enter] The c program will show answer 50 */ #include<stdio.h> int main() { char operator; float num1, num2, result; /* Input a number operator and second number from user */ printf("Enter firrst number operaor second number[ENTER]:"); printf("\nExample: 10+20[Enter]: "); scanf("%f%c%f", &num1,&operator,&num2); /* check the operator and perform operation accordingly */ if( operator=='+') { result= num1+num2; printf("Addition=%.2f",result); } else if (operator =='-') { result = num1- num2; printf("Subtraction=%.2f",result); } else if (operator =='*') { result = num1 * num2; printf("Multiplication=%.2f",result); } else if (operator =='/') { result = num1 / num2; printf("Division=%.2f",result); } else printf("Invalid operator please input +, -, *, / ") ; return 0; }