Topic: Python Tutorial File Handling Programs
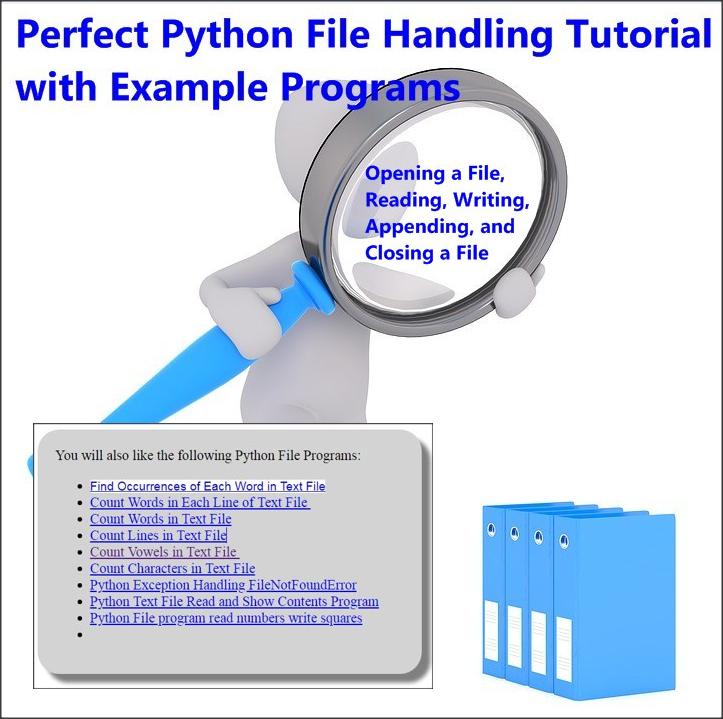
Python File Handling Tutorial With Example Programs
The Python File Programs Covered in this Tutorial:
- Python Program To Maintain a Phone Book / address book to keep track of your friends phone numbers and e-mail addresses using Python Files [Link at bottom of this Page]
Python Tutorial File Handling Programs More than 10 Programs
-
Find Occurrences of Each Word in Text File
-
Count Words in Each Line of Text File
-
Count Words in Text File
-
Count Lines in Text File
-
Count Vowels in Text File
-
Count Characters in Text File
-
Python Exception Handling FileNotFoundError
-
Python Text File Read and Show Contents Program
-
Python File program read numbers write squares
-
Python File Copy Program using shutil.filecopy
-
Python Count Each Vowel in Text File Program
File I/O – File Handling in Python
File handling in Python is an important programming topic. Because we can use the files to store data on hard disk permanently.
The Basic File Operations on Files in Python
1. Opening a File
2. Closing a file
3. Reading a File
4. Writing into a File
Normal Flow of File Operations in a Python Program
1. Open a file
First of all, we will open a file to perform diffetent operations on it.
2. Perform any Operations
Here, we will perform required operations on the file for example, reading, traversing, or writing etc.
Read or write (perform operation)
3. Close the file
After completing the operations on the opened file, it is important to close the file.
How to perform open operation on a file in Python?
Python provides a predefined function open() to open a file. This function will take an argument which is filename and path to open the file. If we do not
specify a path, the default path of the file is in current folder / directory. The open() functions returns a file handle object to access and use the file in the
program.
Syntax of open() function with Example
open() function will take two arguments, a filename possibly with path and a file opening mode.
Example: open a file named “example.txt” from current folder in reading mode.
file1 = open(“example.txt”, “r”)
Example: open a file named “student.txt” from d drive in reading mode.
file2 = open(“student.txt”, “r”)
File Opening Modes in Python
We will provide a second argument to open() function which is a character to specify opening mode.
For example:
We will use “r” file opening mode for file reading
We will use “w” for write operation
We will specify “a” for appending the file.
How To Use Binary File and Text File in Python
We also specify if we want to open the file in text mode or binary mode.
The default is reading in text mode. In this mode, we get strings when reading from the file.
On the other hand, binary mode returns bytes and this is the mode to be used when dealing with non-text files like image or exe files.
Explain Different File Opening Modes in Python
‘r’ is used open a file for reading. It is the default mode.
‘w’ It will open a file for writing. Note: It will creates a new file if it does not exist. It is very dangerous to open an already exited file
with “w” mode, because it will overwrite or truncate the file if it exists. In this case, the data in the file will be lost.
‘x’ “x” is used to open a file for exclusive creation. If the file already exists, the operation fails.
‘a’ “a” stands for append. It opens the given file for appending (any current new data) at the end of the file without truncating it. The append
mode will creates a new file if it does not exist.
‘+’ Open a file for reading and writing. A file including “+” in file opening mode may perform both functions of reading and writing.
Open file in Binary Mode or Text Mode
‘t’ “t” stands for text mode. It is a deafault file opening mode. It will open a file in text mode.
‘b’ “b” stands for binary. It will open a binary file.
Examples of using File Opening Modes
file1 = open(“sample.txt”)
or
file1 = open(“sample.txt”, “r”)
or
file1 = open(“sample.txt”, “rt”)
f1 = open(“abc.txt”,’w’) # open text file for writing
f2 = open(“test.txt”,’a’) # open text file for appending
f = open(“pic.bmp”,’rb’) # open binary file for reading
f = open(“pic.jpg’,’r+b’) # open for reading and writing in binary file
Using close() method to close a file in Python
Properly closing of an opened file, after performing the required operations is very important. The close() method is used with the file object to close a file
properly and free the resources assigned to that file.
The Syntax of close() Method in Python
file1.close() # It will close the file attached with file1 file object/ file handle.
Performing a write Operation to File in Python
The write() method is used to write data in the file. We will use write() method to write a string in a text file or write a sequence of bytes in binary file.
method. This method returns the number of characters written to the file.
For example to write these three lines of text in a file “sample.txt”, we use:
file1 = open(“sample.txt”, “w”)
file1.write(“Line number1\nLine number 2\nLine number 3\n”)
file1.close()
The above Python code / program will create a new file named ‘sample.txt’ if it does not exist. If it exists already, it is overwritten / truncated.
Writing a string or sequence of bytes (for binary files) is done using write() method. This method returns the number of characters written to the file.
How to read data from opened files in Python?
We can use a read() method to read file contents in Python. We will open a file in reading mode, so that we can use read() method for reading file data.
Examples of read() Method
We can use a read(n) method wehere n is the size of file data to be read in one time. If we do not specify size n, the read() method will read the all data of
file.
# read all data written sample.txt file by the code above, and display it
file1 = open(“sample.txt”, “r”)
data = file1.read()
print(data)
The output will be:
Line number1
Line number 2
Line number 3
Example of read(size)
# write three lines in a file
file1 = open(“sample.txt”, “w”)
file1.write(“Line number1\nLine number 2\nLine number 3\n”)
file1.close()
# read all data with read method and display it
file1 = open(“sample.txt”, “r”)
data = file1.read(4) # read only 4 char
print(data)
Output:
Line
How to Use readline() method in Python?
We can use readline() method to read a line of file at a time.
Example:
# write three lines in a file
file1 = open(“sample.txt”, “w”)
file1.write(“Line number1\nLine number 2\nLine number 3\n”)
file1.close()
# read all data with read method and display it
file1 = open(“sample.txt”, “r”)
data = file1.readline() # read first line upto \n including
print(data)
Output:
Line number1
How to use readlines() method to read a file in Python?
The readlines() method returns a list of all / remaining lines present in the whole file.
Example of readlnes() method
# write three lines in a file
file1 = open(“sample.txt”, “w”)
file1.write(“Line number1\nLine number 2\nLine number 3\n”)
file1.close()
# read all data with read method and display it
file1 = open(“sample.txt”, “r”)
data = file1.readlines() # returns list of all lines in the file
print(data)
Output:
[‘Line number1\n’, ‘Line number 2\n’, ‘Line number 3\n’]
How to print the List returned by readlines() method line by line?
Example:
# write three lines in a file
file1 = open(“sample.txt”, “w”)
file1.write(“Line number1\nLine number 2\nLine number 3\n”)
file1.close()
# read all data with read method and display it
file1 = open(“sample.txt”, “r”)
data = file1.readlines() # returns list of all lines in the file
print(data)
# use the for loop to print list data line by line
for line in data:
print(line, end=”)
Output:
[‘Line number1\n’, ‘Line number 2\n’, ‘Line number 3\n’]
Line number1
Line number 2
Line number 3
How To Read a File Line by Line using for Loop in Python?
We can read a file line-by-line using a for loop as shown as under:
for line in fie1:
print(line, end = ”)
Python Complete Program Example:
# write three lines in a file
file1 = open(“sample.txt”, “w”)
file1.write(“Line number1\nLine number 2\nLine number 3\n”)
file1.close()
# read all data with read method and display it
file1 = open(“sample.txt”, “r”)
for line in file1:
print(line, end=”)
Output:
Line number1
Line number 2
Line number 3
How To Copy a File – Python Program
Topic: Python File Copy Program using shutil.filecopy
You can easily copy a source file to a destination file. For this purpose, perform the following steps:
- import shutil module
- input source file name
- input destination file name
- call shutil.copyfile(sourcefile,destinationfile)
The Copy File Code in Python
import shutil
source=input(“Enter source file name and path:\n”)
dest=input(“Enter destination file name and path:\n”)
s=shutil.copyfile(source,dest)
print(” The file ‘ ” +source +” ‘ copied to ‘” + s + ” ‘ successfully”)
Python Tutorial File Handling Programs
You will also like the following Python File Programs:
- Python Program To Maintain a Phone Book / address book to keep track of your friends phone numbers and e-mail addresses using Python Files
-
Find Occurrences of Each Word in Text File
-
Count Words in Each Line of Text File
-
Count Words in Text File
-
Count Lines in Text File
-
Count Vowels in Text File
-
Count Characters in Text File
-
Python Exception Handling FileNotFoundError
-
Python Text File Read and Show Contents Program
-
Python File program read numbers write squares
-
Python File Copy Program using shutil.filecopy
-
Python Count Each Vowel in Text File Program